Augmented Reality with ARKit: Building AR Experiences in iOS Apps
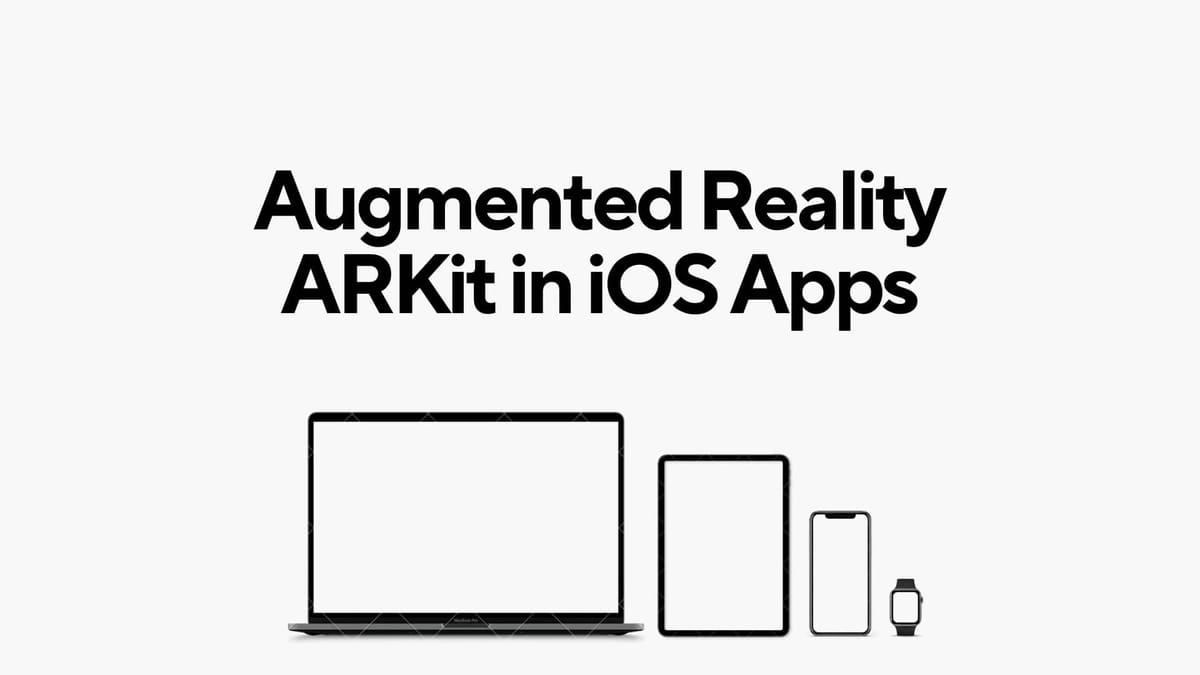
Augmented Reality (AR) has transformed the way we interact with the digital world, and Apple's ARKit empowers iOS developers to seamlessly integrate immersive AR experiences into their apps. In this step-by-step tutorial, we will explore the capabilities of ARKit and guide you through the process of building captivating AR experiences for iOS applications.
Introduction to ARKit
ARKit, Apple's AR framework, provides developers with the tools to blend digital content with the real world, creating unparalleled AR experiences on iOS devices. With features like world tracking, scene understanding, and light estimation, ARKit offers a robust foundation for building interactive and dynamic AR applications.
Setting Up Your Project
- Create a New Xcode Project:
Start by launching Xcode and creating a new project. Choose a template that fits your app's structure, such as a Single View App. - Enable ARKit:
In your project settings, go to the "Signing & Capabilities" tab. Enable the "ARKit" capability to ensure that your app has access to the ARKit framework.
ARKit Essentials: Session Configuration
ARKit operates through an AR session, which manages the motion and scene tracking. Configure your AR session by adding the necessary properties to your view controller.
import ARKit
class ARViewController: UIViewController, ARSCNViewDelegate {
// Create an AR session
let arSession = ARSession()
// Configure the AR scene view
lazy var arSceneView: ARSCNView = {
let sceneView = ARSCNView()
sceneView.session = arSession
sceneView.delegate = self
return sceneView
}()
override func viewDidLoad() {
super.viewDidLoad()
// Set up and add the AR scene view to your view hierarchy
// ...
// Run the AR session
arSession.run(ARWorldTrackingConfiguration())
}
}
Adding Virtual Objects to the AR Scene
Let's enhance our AR experience by adding virtual objects to the scene. For simplicity, we'll add a 3D cube.
func addVirtualObject() {
// Create a 3D cube
let cube = SCNBox(width: 0.1, height: 0.1, length: 0.1, chamferRadius: 0.0)
let cubeNode = SCNNode(geometry: cube)
// Position the cube in front of the camera
if let cameraTransform = arSession.currentFrame?.camera.transform {
var translation = matrix_identity_float4x4
translation.columns.3.z = -0.5
cubeNode.simdTransform = matrix_multiply(cameraTransform, translation)
}
// Add the cube to the AR scene
arSceneView.scene.rootNode.addChildNode(cubeNode)
}
Interacting with AR Objects: Adding Gestures
Enhance user interaction by adding gestures to manipulate AR objects. In this example, we'll implement a tap gesture to move the virtual cube.
func addTapGesture() {
let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap(_:)))
arSceneView.addGestureRecognizer(tapGesture)
}
@objc func handleTap(_ gesture: UITapGestureRecognizer) {
let tapLocation = gesture.location(in: arSceneView)
// Perform a hit test to identify AR objects at the tap location
let hitTestResults = arSceneView.hitTest(tapLocation, types: .existingPlaneUsingExtent)
// If a hit is found, move the virtual object to the hit location
if let hitResult = hitTestResults.first {
SCNTransaction.begin()
SCNTransaction.animationDuration = 0.5
virtualObjectNode.position = SCNVector3(hitResult.worldTransform.columns.3.x,
hitResult.worldTransform.columns.3.y,
hitResult.worldTransform.columns.3.z)
SCNTransaction.commit()
}
}
Enhancing Realism with Lighting
To enhance the realism of your AR scene, leverage ARKit's light estimation capabilities. Adjust the lighting of your virtual objects based on the ambient lighting conditions.
func renderer(_ renderer: SCNSceneRenderer, didUpdate node: SCNNode, for anchor: ARAnchor) {
// Check if the anchor has lighting information
if let lightEstimation = (arSceneView.session.currentFrame?.lightEstimate) {
// Adjust the lighting of virtual objects based on the estimated environment lighting
node.childNodes.forEach { childNode in
if let object = childNode.geometry {
object.materials.forEach { material in
material.lightingModel = .physicallyBased
material.diffuse.contents = UIColor(white: CGFloat(lightEstimation.ambientIntensity), alpha: 1.0)
}
}
}
}
}
Building AR Experiences with ARKit
In conclusion, ARKit empowers iOS developers to create immersive AR experiences with ease. From setting up your project to adding virtual objects and implementing gestures, this tutorial provides a solid foundation for building AR applications on iOS. As you continue your exploration of ARKit, consider incorporating additional features like plane detection, object recognition, and spatial audio to create even more engaging and interactive AR experiences. With ARKit's powerful capabilities at your fingertips, the possibilities for innovation within the iOS ecosystem are boundless. Happy coding, and may your AR endeavors bring a new dimension to the world of iOS development!