Closures in Swift
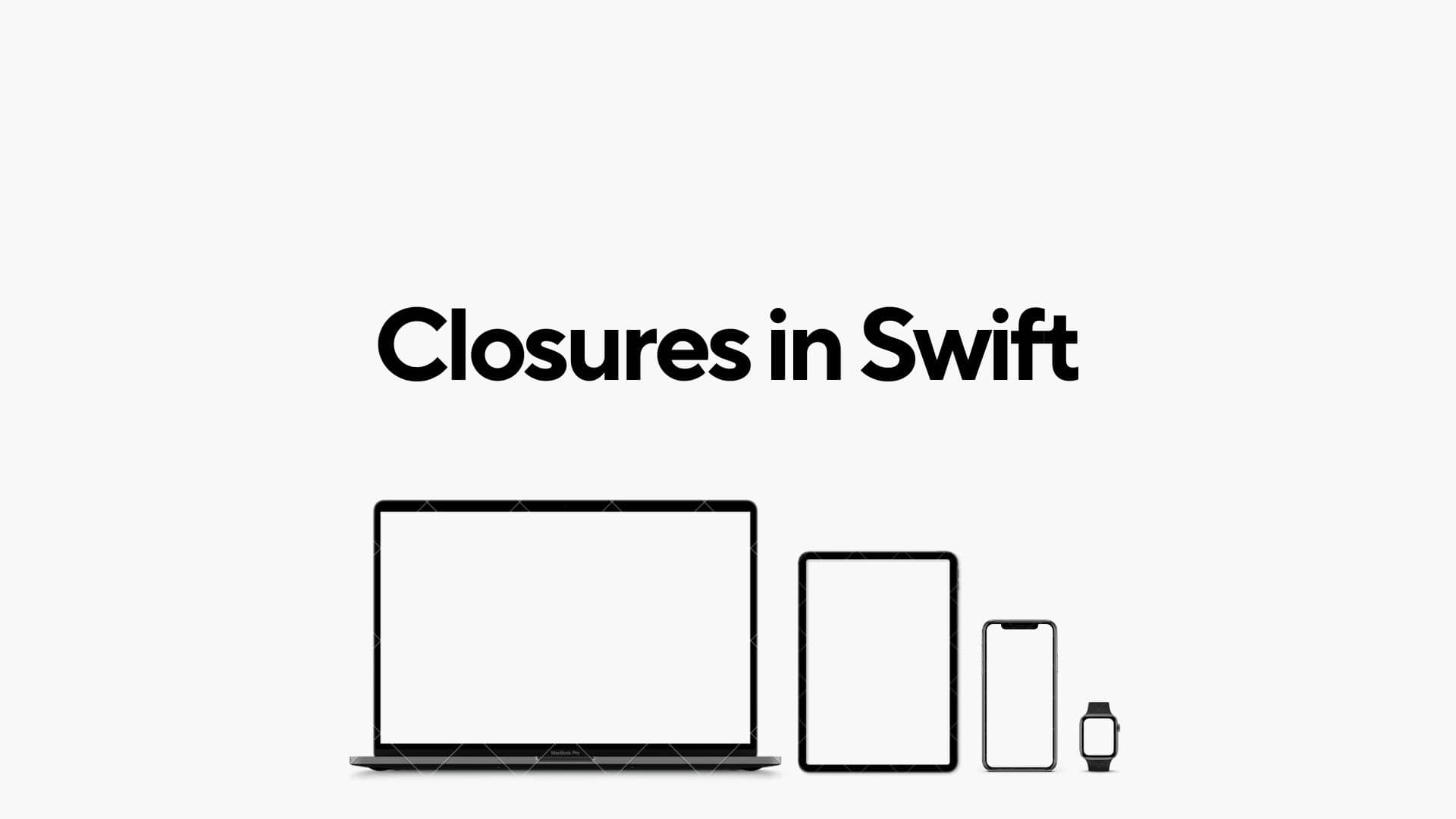
Closures in Swift are not just anonymous functions; they're self-contained blocks of code that can capture values from their surrounding environment, allowing you to write concise, flexible, and expressive code. Whether you're new to Swift or looking to deepen your understanding, this guide will dive into the fundamentals and practical applications of closures.
What are Closures in Swift?
Closures are self-contained blocks of functionality that can be passed around and used in Swift code. They capture and store references to any constants and variables from the context in which they are defined. Closures are a fundamental part of Swift programming and are commonly used for tasks such as sorting collections, performing asynchronous operations, and implementing functional programming patterns.
Imagine a miniature, portable function that can be passed around and executed on demand. That's essentially what a closure is. Defined using curly braces {}
, it can encapsulate logic without requiring a separate named function. This makes closures ideal for:
- Passing code as arguments: Send closures to functions as arguments to create custom behavior, like sorting or filtering data.
- Asynchronous operations: Use closures with completion handlers to manage tasks that take time to complete, ensuring your code doesn't block.
- Event handling: Attach closures to UI elements to respond to user interactions dynamically.
- Customizing algorithms: Craft closures to tailor built-in functions like
map
,reduce
, andfilter
to your specific needs.
Syntax and Types:
let greet = {
print("Hello, world!")
}
greet() // Output: Hello, world!
In this example, we define a closure named greet
that takes no parameters and prints "Hello, world!" when called.
Let's break down the closure syntax:
{ parameters in return type -> code }
- Parameters (optional): Define any values the closure receives.
in
: Marks the start of the closure body.- Return type (optional): Specifies the type of value the closure returns.
- Code: Contains the actual logic to be executed.
To work with closures effectively, grasp the concept of types:
- Closure types: Specify the expected behavior and compatibility of closures. Define them like function types:
(Int, String) -> Bool
. - Capturing values: Closures can access variables and constants from their surrounding scope (environment). This is known as "closure capture."
Common Use Cases:
Now, let's explore some real-world scenarios where closures shine:
- Sorting data:
let numbers = [5, 3, 1, 8, 2]
let sortedNumbers = numbers.sorted(by: { $0 < $1 }) // Closure sorts in ascending order
- Filtering items:
let evenNumbers = numbers.filter { $0 % 2 == 0 } // Closure filters even numbers
- Responding to button clicks:
button.addTarget(self, action: { [weak self] in
self?.dismissViewController(animated: true) // Closure dismisses the view controller
}, for: .touchUpInside)
- Asynchronous network requests:
URLSession.shared.dataTask(with: url) { data, response, error in
if let error = error {
print(error.localizedDescription)
} else if let data = data {
// Process data in the closure
}
}.resume()
Beyond the Basics:
While these examples showcase the core functionality, closures offer even more power when you:
- Capture values by reference or value: Understand the nuance of capturing values to avoid unintended side effects.
- Handle escaping closures: Employ the
@escaping
attribute to ensure closures are properly handled across function boundaries. - Leverage trailing closures: Simplify syntax for closures passed as the last argument to a function.
- Embrace autoclosures: Use the
@autoclosure
attribute for concise closure expressions.
Using Closures:
Closures can be assigned to variables, passed as arguments to functions, and returned from functions, just like any other value in Swift. Let's see how we can use closures in practice:
let numbers = [1, 2, 3, 4, 5]
let mappedNumbers = numbers.map { $0 * 2 }
print(mappedNumbers) // Output: [2, 4, 6, 8, 10]
In this example, we use the map
function to apply a closure that multiplies each element of the numbers
array by 2. The closure { $0 * 2 }
is passed as an argument to the map
function and is applied to each element of the array.
Capturing Values in Closures
Closures can capture and store references to any constants and variables from the context in which they are defined. This means that they can access and modify variables that are defined outside of their own scope. Let's look at an example:
func makeCounter() -> () -> Int {
var count = 0
return {
count += 1
return count
}
}
let counter = makeCounter()
print(counter()) // Output: 1
print(counter()) // Output: 2
print(counter()) // Output: 3
In this example, the makeCounter
function returns a closure that captures and modifies the count
variable defined in its outer scope. Each time the closure is called, it increments the count
variable and returns its current value.
Trailing Closures
In Swift, if a closure is the last argument to a function, you can use trailing closure syntax for a cleaner and more readable code. Let's see an example:
let numbers = [1, 2, 3, 4, 5]
let sum = numbers.reduce(0) { $0 + $1 }
print(sum) // Output: 15
In this example, we use the reduce
function with a trailing closure to calculate the sum of all elements in the numbers
array.
Closures are a fundamental tool in every Swift developer's arsenal. By mastering their concepts and applications, you can write cleaner, more flexible, and maintainable code, unlocking a new level of expressiveness in your iOS projects. Remember, practice, experiment, and don't hesitate to seek help when needed. Happy coding!