Guard with Optionals in Swift
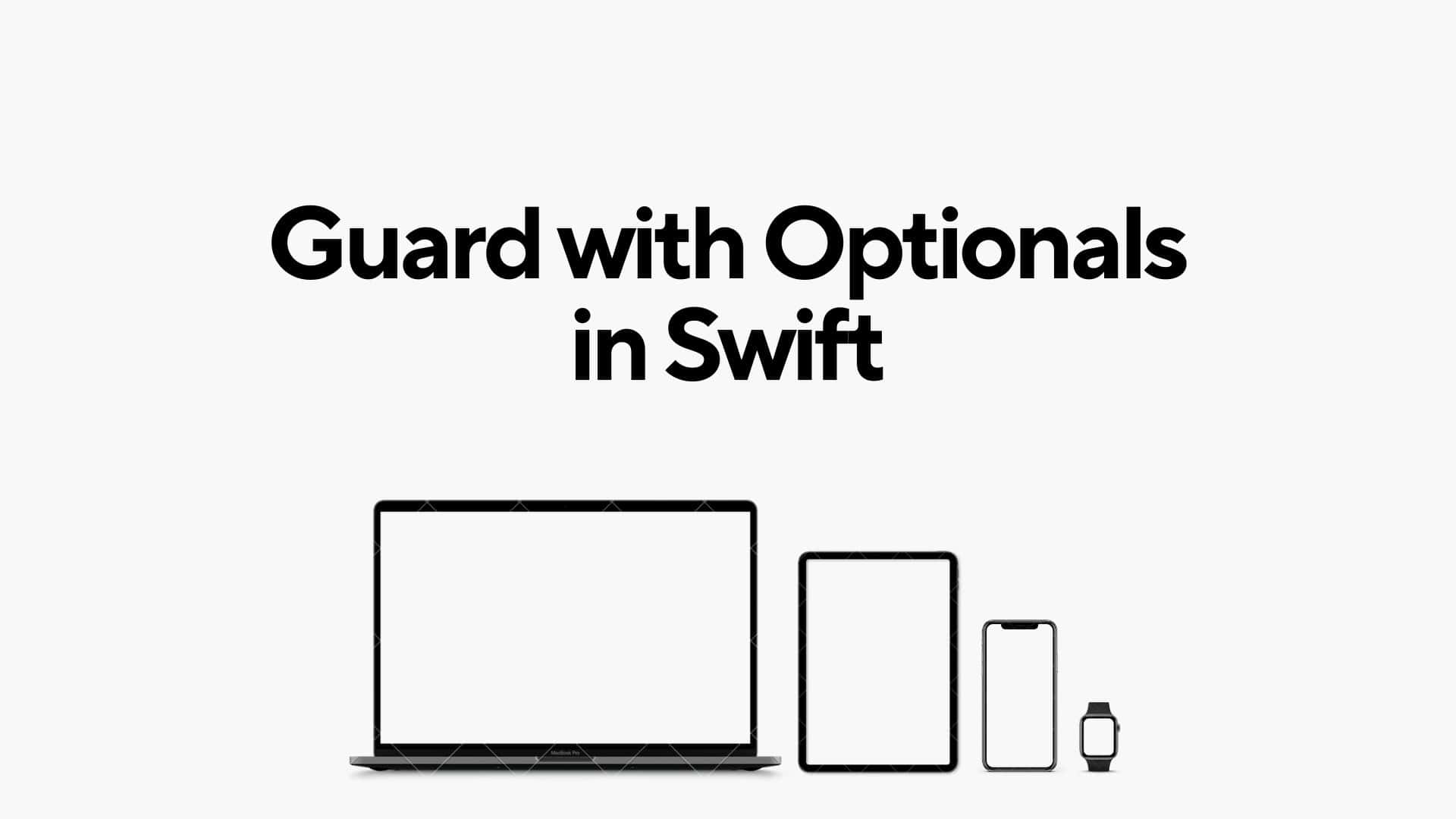
In Swift, guard
statements are commonly used with optionals to handle early exits from a function or block of code if certain conditions are not met. The guard
statement provides a clean and readable way to unwrap optionals and handle potential failure cases without deeply nesting your code.
Using guard
with Optionals
The guard
statement is used to check if a condition is true. If the condition is false, it must exit the current scope using a return
, break
, continue
, or throw
statement. This makes it especially useful for optional unwrapping, ensuring that certain values are available before proceeding.
Here's a basic example:
func printName(name: String?) {
guard let unwrappedName = name else {
print("Name is nil")
return
}
print("The name is \(unwrappedName)")
}
In this example:
- The
guard
statement checks if thename
optional contains a value. - If
name
isnil
, the code inside theelse
block is executed, which prints "Name is nil" and exits the function. - If
name
contains a value, it is unwrapped and assigned tounwrappedName
, and the code proceeds to print the name.
Benefits of Using guard
with Optionals
- Readability:
guard
helps keep code more readable by avoiding deep nesting. Conditions that would cause an early exit are handled upfront, making the main logic of the function easier to follow. - Safety: Using
guard
ensures that required conditions are met before proceeding, reducing the risk of runtime errors due to nil values or invalid states. - Clarity: It clearly communicates the intent to exit early if conditions are not met, making the code easier to understand and maintain.
Example: Function with Multiple guard
Statements
You can use multiple guard
statements to check various conditions. Here’s an example where a function processes user information, requiring both a valid name and age:
struct User {
var name: String?
var age: Int?
}
func processUser(user: User) {
guard let name = user.name else {
print("User name is missing")
return
}
guard let age = user.age, age >= 18 else {
print("User must be at least 18 years old")
return
}
print("Processing user \(name), age \(age)")
}
let user1 = User(name: "Alice", age: 22)
let user2 = User(name: nil, age: 22)
let user3 = User(name: "Bob", age: 17)
processUser(user: user1) // Outputs: Processing user Alice, age 22
processUser(user: user2) // Outputs: User name is missing
processUser(user: user3) // Outputs: User must be at least 18 years old
In this example:
- The
guard
statement first checks if the user’s name is not nil. - The second
guard
statement checks if the user’s age is not nil and if the user is at least 18 years old. - If any condition fails, the function exits early, and an appropriate message is printed.
- If all conditions are met, the function processes the user.
Combining guard
with Other Optional Handling Techniques
While guard
is powerful, it’s often used alongside other optional handling techniques like optional chaining and nil coalescing to write concise and safe code. Here’s an example combining these techniques:
func greet(user: User?) {
guard let user = user else {
print("User is nil")
return
}
guard let name = user.name, let age = user.age, age >= 18 else {
print("Invalid user information")
return
}
print("Hello, \(name), age \(age)")
}
let optionalUser: User? = User(name: "Charlie", age: 20)
greet(user: optionalUser) // Outputs: Hello, Charlie, age 20
In this example:
- The first
guard
statement ensures theuser
is not nil. - The second
guard
statement ensures thename
andage
properties are not nil and that the user is at least 18 years old. - If all conditions are met, the greeting message is printed.
Conclusion
Using guard
with optionals in Swift is a powerful technique for writing clean, readable, and safe code. It allows you to handle early exits and ensure that necessary conditions are met before proceeding with the main logic of your function. By leveraging guard
, you can avoid deep nesting and make your code easier to understand and maintain.