How to use Return keyword in Swift functions?
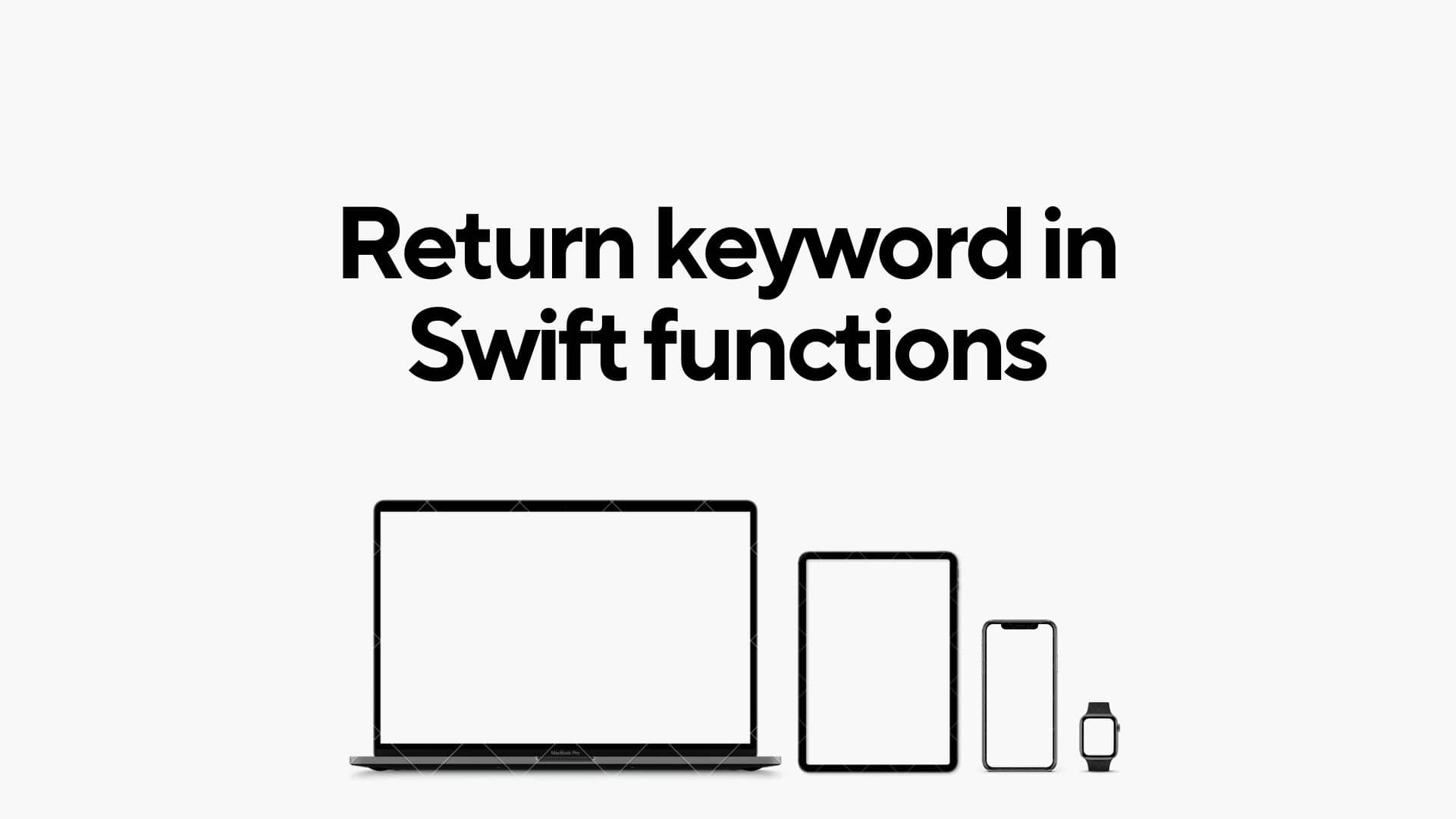
In Swift, the return
keyword is used within functions to indicate the value that the function should return when it's called. Whether the function returns a single value, multiple values (using tuples), or no value at all (void), the return
statement plays a crucial role in specifying the output of the function. Let's explore how the return
keyword is used in different scenarios within Swift functions:
1. Returning a Single Value
func add(a: Int, b: Int) -> Int {
return a + b
}
let result = add(a: 3, b: 5)
print("Result: \(result)") // Output: Result: 8
In this example, the function add
takes two parameters a
and b
, both of type Int
, and returns their sum as an Int
value using the return
keyword.
2. Returning Multiple Values using Tuples
func minMax(array: [Int]) -> (min: Int, max: Int)? {
if array.isEmpty { return nil }
var currentMin = array[0]
var currentMax = array[0]
for value in array[1..<array.count] {
if value < currentMin {
currentMin = value
} else if value > currentMax {
currentMax = value
}
}
return (currentMin, currentMax)
}
let numbers = [10, 5, 3, 15, 7]
if let bounds = minMax(array: numbers) {
print("Min: \(bounds.min), Max: \(bounds.max)") // Output: Min: 3, Max: 15
}
In this example, the function minMax
takes an array of integers as input and returns a tuple containing the minimum and maximum values found in the array. The return
keyword is used to return the tuple from the function.
3. Returning Void (No Value)
func greet(name: String) {
print("Hello, \(name)!")
}
greet(name: "Alice") // Output: Hello, Alice!
In this example, the function greet
takes a name
parameter of type String
and returns no value (void). The return
keyword is used implicitly to indicate the end of the function execution, and no value needs to be explicitly returned.
4. Early Return in Functions
func divide(a: Int, by b: Int) -> Int? {
guard b != 0 else {
return nil // Return early if division by zero is attempted
}
return a / b
}
if let result = divide(a: 10, by: 2) {
print("Result: \(result)") // Output: Result: 5
}
In this example, the function divide
takes two parameters a
and b
, both of type Int
, and returns the result of dividing a
by b
. However, it checks for the condition b != 0
using a guard
statement and returns nil
early if division by zero is attempted, using the return
keyword within the guard
block.
return keyword in Swift
The return
keyword in Swift functions is a fundamental tool for specifying the output of a function, whether it's a single value, multiple values using tuples, or no value at all (void). Understanding how to use the return
keyword effectively allows Swift developers to create more expressive, flexible, and robust functions in their code.