Initializer and Deinitializer in Swift: Instance Lifecycle
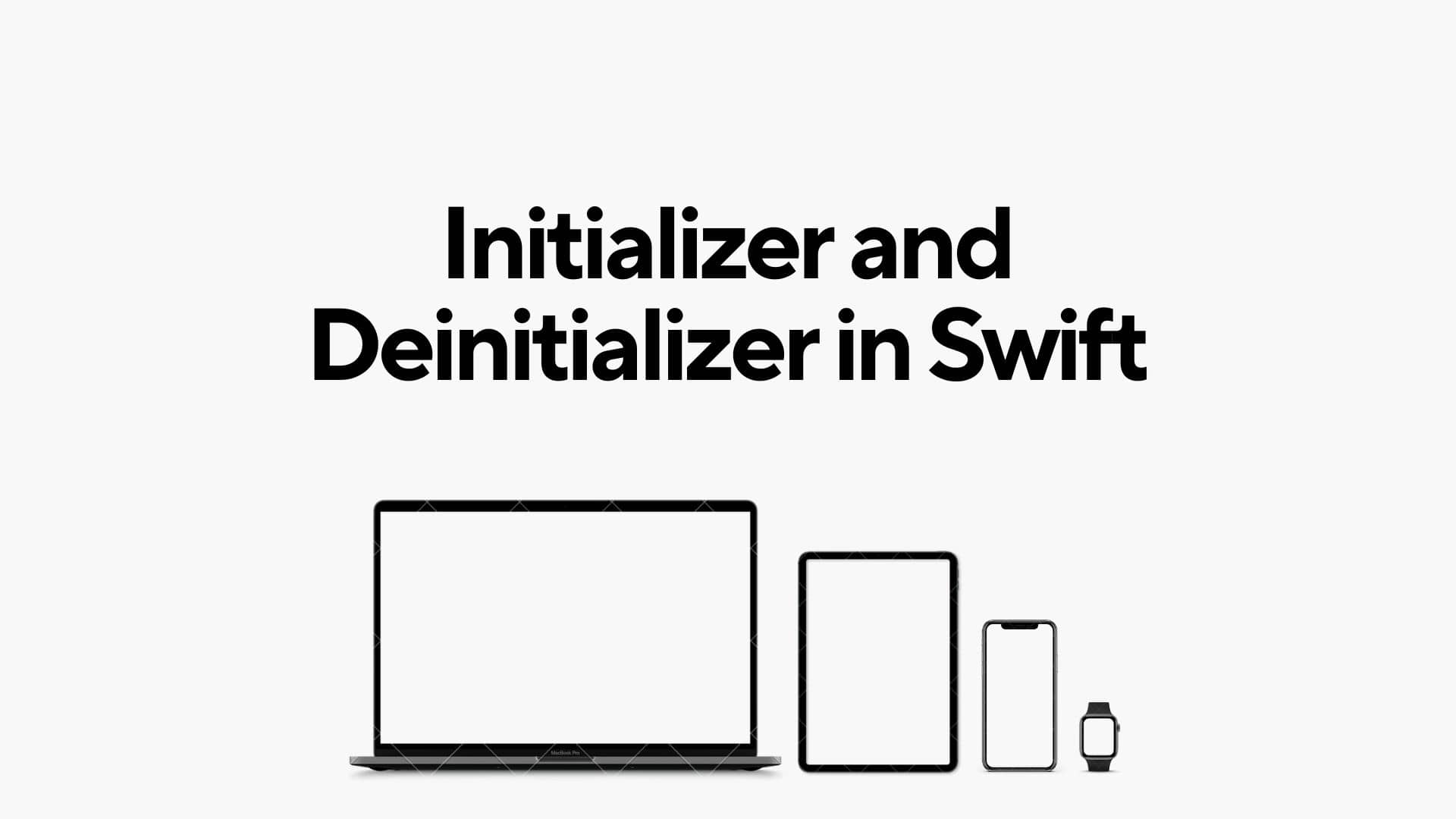
In Swift, initializers and deinitializers are essential components for managing the lifecycle of instances of classes, structs, and enumerations. They are responsible for initializing and deinitializing the properties and resources associated with an instance. Let's explore each of them in detail:
Initializers:
Initializers are special methods that are called to create a new instance of a class, struct, or enumeration. They initialize the properties and perform any necessary setup to prepare the instance for use. There are several types of initializers:
- Designated Initializers: These are the primary initializers for a class, struct, or enumeration. They fully initialize all properties introduced by that class and call an appropriate superclass initializer if the class is a subclass.
class Person {
var name: String
init(name: String) {
self.name = name
}
}
- Convenience Initializers: These are secondary initializers that provide additional initialization options. They must call a designated initializer of the same class.
class Person {
var name: String
init(name: String) {
self.name = name
}
convenience init() {
self.init(name: "Unknown")
}
}
- Failable Initializers: These initializers can fail to initialize an instance and return
nil
. They are denoted by placing a?
or!
afterinit
.
class Person {
var name: String
init?(name: String) {
if name.isEmpty {
return nil
}
self.name = name
}
}
Deinitializers:
Deinitializers are special methods that are called when an instance of a class is deallocated from memory. They perform cleanup tasks and release any resources associated with the instance. Deinitializers are defined using the deinit
keyword.
class File {
var fileName: String
init(fileName: String) {
self.fileName = fileName
}
deinit {
// Perform cleanup tasks
print("File \(fileName) is being deallocated")
}
}
Deinitializers are called automatically by the Swift runtime when an instance is no longer referenced or needed, and its memory is reclaimed.
Automatic Reference Counting (ARC):
Swift uses Automatic Reference Counting (ARC) to manage memory and automatically deallocate instances when they are no longer needed. ARC tracks the number of references to an instance and deallocates it when the reference count drops to zero.
var file1: File? = File(fileName: "document.txt")
var file2: File? = file1
file1 = nil // Decrements reference count
file2 = nil // Deallocates the File instance
In this example, the File
instance is deallocated when both file1
and file2
are set to nil
, causing the reference count to drop to zero.
Takeaway:
Initializers and deinitializers are critical for managing the lifecycle of instances in Swift. They allow you to initialize properties, perform setup tasks, and release resources when instances are no longer needed. Understanding how to define and use initializers and deinitializers is essential for writing safe and efficient Swift code, especially when working with classes and managing memory.