Introduction to SwiftUI - Design iOS Apps
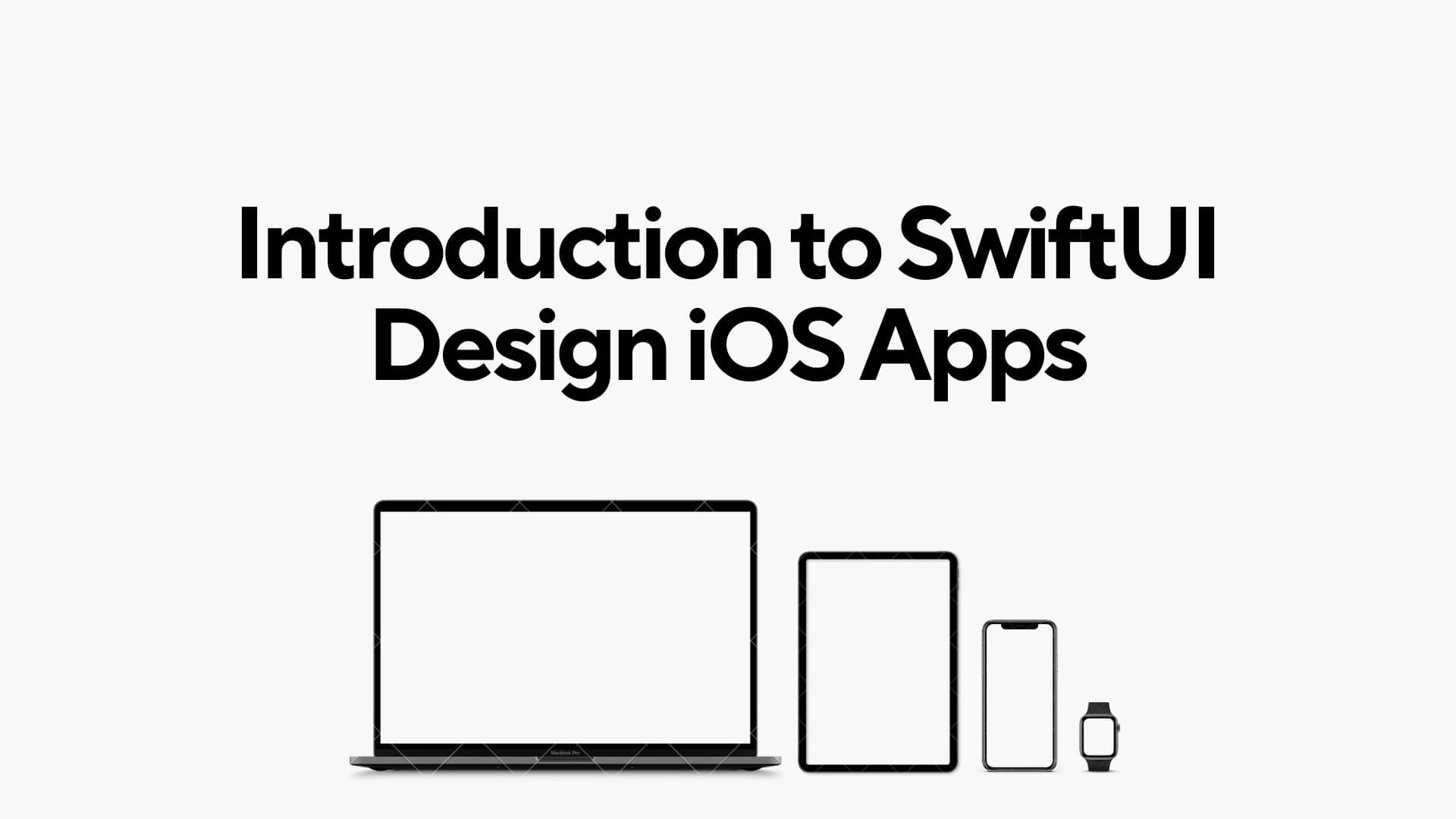
SwiftUI is a user interface toolkit developed by Apple for building user interfaces across all Apple platforms, including iOS, macOS, watchOS, and tvOS. It was introduced in 2019 and is designed to be easy to use, flexible, and powerful. SwiftUI is built using Swift, Apple's programming language.
Here's an overview of key concepts and features in SwiftUI:
- Declarative Syntax:
SwiftUI uses a declarative syntax, meaning you describe the user interface's appearance and behavior, and the framework takes care of the underlying implementation. This is in contrast to the imperative approach where you explicitly specify step-by-step instructions. - Views:
In SwiftUI, everything is a view. Views are the basic building blocks of your user interface. They can represent simple things like text or images, or complex structures like lists, forms, and navigation stacks. - Modifiers:
Modifiers are used to change the appearance or behavior of views. You apply modifiers to views using a chaining syntax. For example, you can use modifiers to change the font, color, or spacing of a text view.
Text("Hello, SwiftUI!")
.font(.title)
.foregroundColor(.blue)
- Containers:
SwiftUI provides various container views to arrange and organize other views. Examples includeVStack
(vertical stack),HStack
(horizontal stack),ZStack
(z-axis stack), andList
for displaying scrollable lists.
VStack {
Text("Hello")
Text("SwiftUI")
}
- State:
State is a crucial concept in SwiftUI for managing the dynamic data of your app. When the state changes, the framework automatically updates the affected views. You can use the@State
property wrapper to declare mutable state within a view.
@State private var counter = 0
var body: some View {
Text("Counter: \(counter)")
.onTapGesture {
counter += 1
}
}
- Binding:
Binding is a way to create a two-way connection between a view and its underlying model. It allows changes in one to automatically update the other.
struct ContentView: View {
@State private var toggleValue = false
var body: some View {
Toggle("Toggle", isOn: $toggleValue)
}
}
- Navigation:
SwiftUI includes navigation views and navigation links for handling navigation within your app. You can create navigation stacks and push views onto the stack as the user navigates through the app.
NavigationView {
NavigationLink(destination: Text("Detail View")) {
Text("Go to Detail")
}
}
- Preview:
SwiftUI comes with a live preview feature in Xcode that allows you to see the changes you make to your code in real-time. This makes the development process more interactive and efficient. - Combine Framework:
SwiftUI integrates with the Combine framework, which is Apple's reactive programming framework. This allows you to handle asynchronous and event-driven code more effectively.
This overview provides a glimpse into the core concepts of SwiftUI. As you delve deeper, you'll discover more features and capabilities that make SwiftUI a powerful and modern framework for building Apple platform applications.