Mutating Methods in Swift
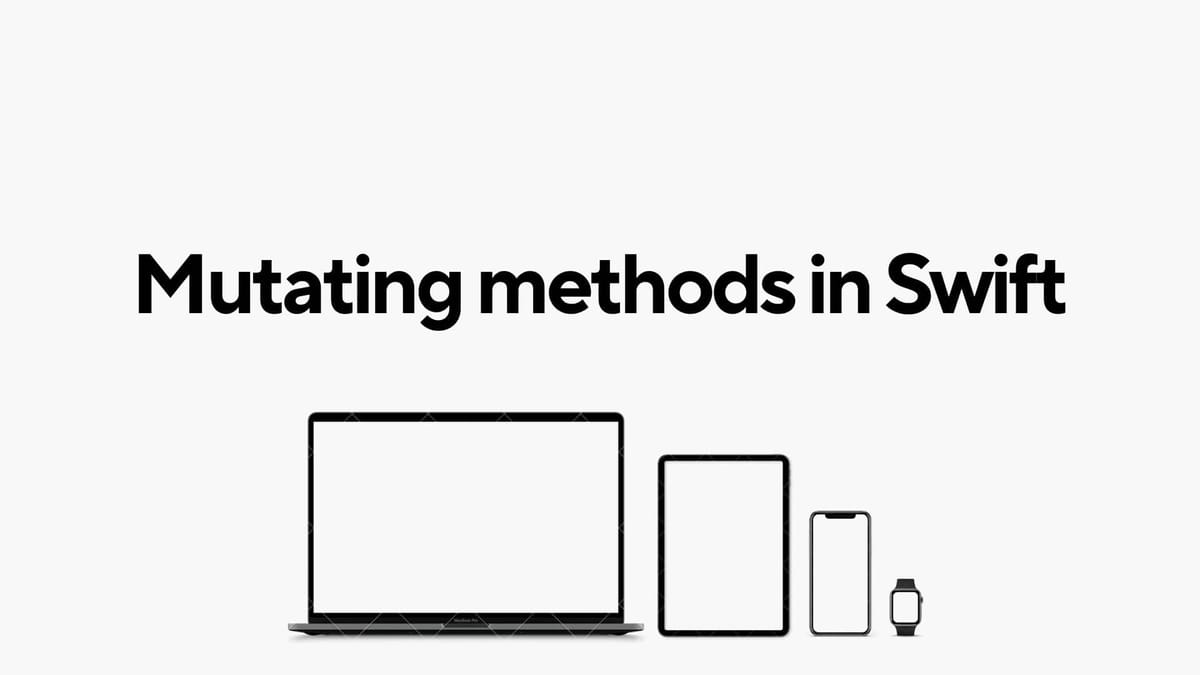
In Swift, structures (and enumerations) are value types. By default, the properties of a value type cannot be modified within its instance methods. However, there are scenarios where you might want to modify the properties of a structure within its instance methods. To facilitate this, Swift provides the mutating
keyword.
1. Understanding mutating
Methods:
The mutating
keyword is used to mark instance methods that modify properties of the structure (or enumeration) they belong to. By marking a method as mutating
, you indicate to the compiler that the method is allowed to modify the properties of the structure.
2. Syntax of mutating
Methods:
Here's the syntax of defining a mutating method in Swift:
struct SomeStruct {
var value: Int
mutating func modifyValue(newValue: Int) {
value = newValue
}
}
In this example, modifyValue
is a mutating method of the SomeStruct
structure. It takes a new value as a parameter and assigns it to the value
property.
3. Using mutating
Methods:
You can call mutating methods on instances of the structure. When calling a mutating method on a variable (not a constant), you must use the var
keyword to declare the variable, indicating that it's mutable.
var instance = SomeStruct(value: 5)
instance.modifyValue(newValue: 10)
print(instance.value) // Output: 10
4. Limitations of mutating
Methods:
- Mutating methods can only be called on instances of the structure (or enumeration) that are declared using the
var
keyword, indicating mutability. - Mutating methods cannot be called on instances declared using the
let
keyword, as constants are immutable.
5. When to Use mutating
Methods:
You should use mutating methods when you need to modify the properties of a structure (or enumeration) from within its instance methods. This is particularly useful when working with value types and you want to mutate their state.
6. Example:
Here's an example demonstrating the use of a mutating method in a structure representing a simple counter:
struct Counter {
var count: Int
mutating func increment() {
count += 1
}
}
var counter = Counter(count: 0)
counter.increment()
print(counter.count) // Output: 1
In this example, the increment
method is marked as mutating
because it modifies the count
property of the Counter
structure.
Conclusion:
mutating
methods in Swift enable structures (and enumerations) to modify their properties from within instance methods. This feature is essential for working with value types in a flexible and expressive manner. By understanding how to use mutating
methods effectively, Swift developers can write clean and concise code while leveraging the benefits of value semantics.