mutating Structs and @Observable Class - Swift
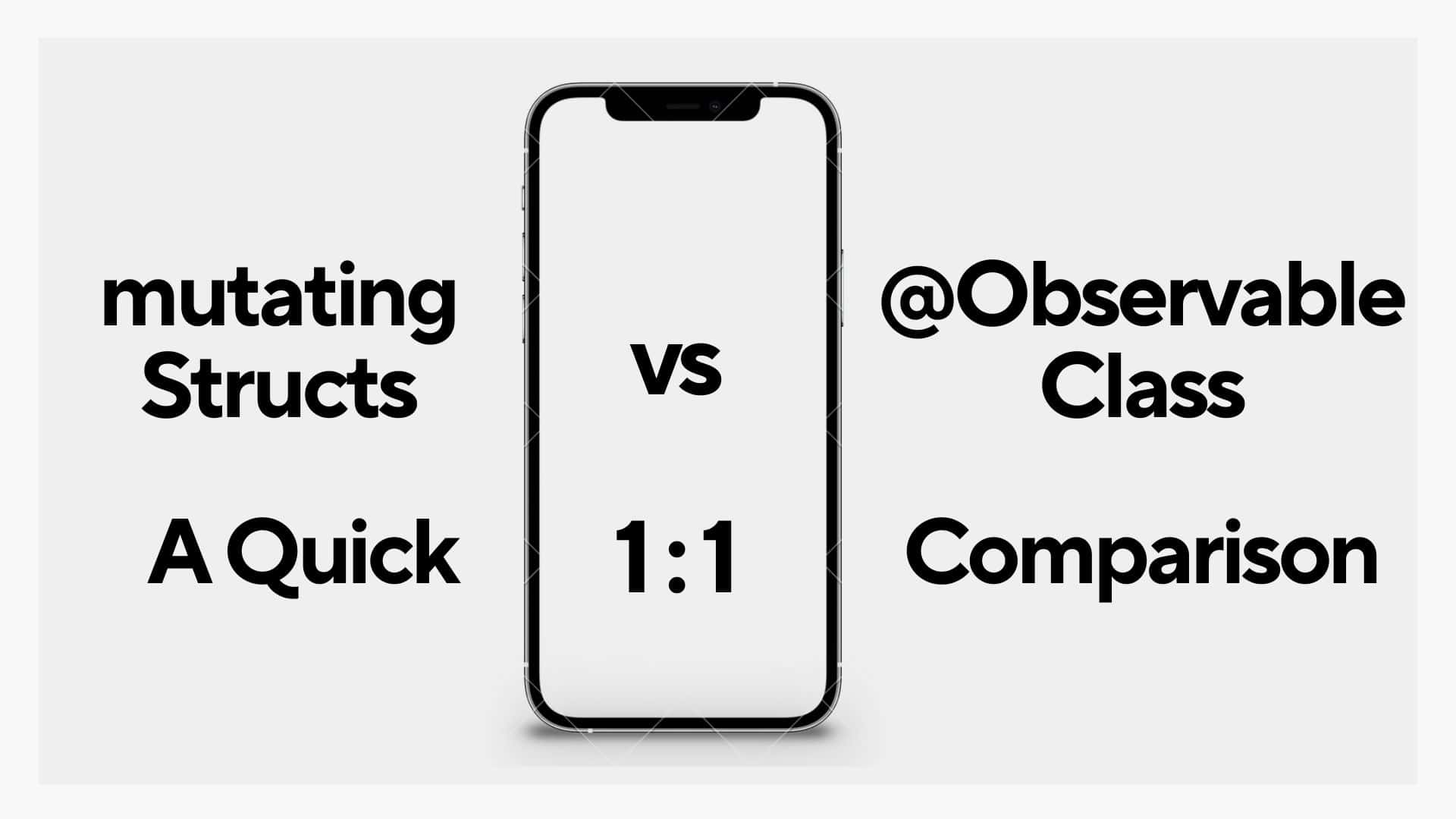
In Swift, you can use both mutating
methods in structs and ObservableObject
classes to manage state, but they serve different purposes and are used in different contexts.
Structs with mutating methods provide a powerful way to work with value types while still allowing for controlled mutability. They are ideal for scenarios where you need to encapsulate data and behavior within lightweight, immutable-by-default data structures.
ObservableObject
provides a powerful mechanism to manage and share state in a SwiftUI app. It simplifies the process of updating the UI in response to data changes, especially when dealing with more complex or shared state scenarios.
Key Differences
- Memory Management:
- Struct: Value type, copied when passed around.
- Class (ObservableObject): Reference type, shared across references.
- UI Updates:
- Struct: Does not automatically notify SwiftUI when changes happen; typically used with
@State
. - ObservableObject: Automatically notifies SwiftUI views of changes, triggering UI updates.
- Struct: Does not automatically notify SwiftUI when changes happen; typically used with
- Mutability:
- Struct: Requires
mutating
keyword for methods that change properties. - Class: Methods can change properties without special keywords.
- Struct: Requires
When to Use
- Use mutating structs when you want to create lightweight, immutable-by-default data structures and you're working within a single scope where you can control mutability explicitly.
- Use ObservableObject classes when you need to manage state across multiple views and want to leverage SwiftUI's automatic view updates.
Mutating Structs
In Swift, structs are value types, meaning each instance keeps its own copy of data. This contrasts with classes, which are reference types where multiple variables can reference the same instance.
By default, properties of a struct cannot be modified within instance methods. However, if you need to modify properties within a method, you can mark that method with the mutating
keyword.
Why Use Structs?
Structs are often preferred for:
- Immutability by Default: Since structs are value types, they are copied when passed around. This makes them naturally thread-safe and ideal for scenarios where you want to ensure that data cannot be modified unexpectedly.
- Performance: For small data types, structs can be more efficient because of their stack allocation and lower overhead compared to heap-allocated classes.
- Simpler State Management: Structs are particularly useful when you're dealing with smaller, well-defined pieces of data, like points, sizes, or custom data structures that you want to ensure remain immutable unless explicitly modified.
Mutating Methods
A mutating
method in a struct allows the method to modify self
, i.e., the instance itself. Here’s how it works:
struct Rectangle {
var width: Double
var height: Double
mutating func scale(by factor: Double) {
width *= factor
height *= factor
}
}
var myRectangle = Rectangle(width: 10, height: 5)
myRectangle.scale(by: 2)
print(myRectangle.width) // Output: 20.0
print(myRectangle.height) // Output: 10.0
In this example, the scale
method modifies the width
and height
properties. Because these properties belong to a struct, and structs are value types, the mutating
keyword is necessary to signal that the method modifies the instance.
Copy-on-Write Semantics
One important aspect of structs in Swift is their copy-on-write behavior. If you assign a struct to a new variable or pass it to a function, a copy is made only if the original or the copy is modified. This ensures efficiency.
var originalRectangle = Rectangle(width: 10, height: 5)
var copyRectangle = originalRectangle
copyRectangle.scale(by: 2)
print(originalRectangle.width) // Output: 10.0
print(copyRectangle.width) // Output: 20.0
Here, originalRectangle
and copyRectangle
are separate instances, and modifying one does not affect the other.
When to Use Structs with Mutating Methods
- Data Encapsulation: When you want to encapsulate behavior that involves modifying internal data within a struct.
- Thread Safety: When passing data between threads, structs ensure that each thread works with its own copy, preventing race conditions.
- Functional Programming Style: Structs align well with functional programming principles where data is immutable by default, and functions are used to produce new versions of data.
Considerations
- Large Data Structures: While structs are efficient for small data types, if a struct holds large amounts of data, copying it can become expensive. In such cases, you might opt for a class.
- Shared State: If you need to share state between different parts of your app, classes might be a better fit since they allow shared references.
ObservableObject Class
ObservableObject
is a protocol in SwiftUI that allows you to create classes whose changes can be observed by the UI. When a property marked with @Published
within an ObservableObject
changes, any views observing the object will automatically update.
In SwiftUI, managing the state of your views is crucial, and ObservableObject
is one of the key tools for doing this. An ObservableObject
is a class that conforms to the ObservableObject
protocol, which allows it to notify any observing views when its properties change. This is particularly useful for handling data that is shared across multiple views or needs to persist beyond the lifetime of a single view.
Why Use ObservableObject?
- Shared State: Unlike structs, which are value types, classes (and therefore
ObservableObject
) are reference types. This allows multiple views to observe and interact with the same instance, making it ideal for shared state. - Automatic UI Updates: When a property marked with
@Published
in anObservableObject
changes, SwiftUI automatically updates any views that are observing the object. This simplifies state management in your app. - Persistent State: Since classes can persist beyond the scope in which they are created,
ObservableObject
is well-suited for managing state that needs to be retained, such as user preferences, game scores, or data fetched from the network.
ObservableObject in Action
Here’s a basic example:
import SwiftUI
class CounterViewModel: ObservableObject {
@Published var count: Int = 0
func increment() {
count += 1
}
func reset() {
count = 0
}
}
struct ContentView: View {
@StateObject private var viewModel = CounterViewModel()
var body: some View {
VStack {
Text("Count: \(viewModel.count)")
Button("Increment") {
viewModel.increment()
}
Button("Reset") {
viewModel.reset()
}
}
}
}
Key Concepts
- @Published: This property wrapper is used within an
ObservableObject
to mark properties that should trigger view updates when they change.
class ExampleViewModel: ObservableObject {
@Published var text: String = "Hello, World!"
}
Anytime text
changes, any view observing this ExampleViewModel
will be re-rendered.
- @StateObject: This is used to create and manage the lifecycle of an
ObservableObject
. It ensures that the view creates the object only once and retains it for the view's lifecycle.
struct ExampleView: View {
@StateObject private var viewModel = ExampleViewModel()
var body: some View {
Text(viewModel.text)
}
}
- @ObservedObject: This is similar to
@StateObject
, but it assumes that theObservableObject
is created elsewhere and is simply being observed by the view. It does not manage the lifecycle of the object.
struct ExampleView: View {
@ObservedObject var viewModel: ExampleViewModel
var body: some View {
Text(viewModel.text)
}
}
- @EnvironmentObject: This is used to share an
ObservableObject
across many views in a SwiftUI app. It allows the object to be injected into the environment, making it accessible in any child view without explicitly passing it.
struct ParentView: View {
@StateObject private var viewModel = ExampleViewModel()
var body: some View {
ChildView()
.environmentObject(viewModel)
}
}
struct ChildView: View {
@EnvironmentObject var viewModel: ExampleViewModel
var body: some View {
Text(viewModel.text)
}
}
When to Use ObservableObject
- Complex State Management: When your app needs to manage more complex or shared state across multiple views.
- Persistent Data: For data that needs to persist beyond the lifetime of a single view or is loaded asynchronously (e.g., data fetched from an API).
- Shared Data: When the same state needs to be observed by multiple views, such as a user’s profile data or settings that affect the entire app.
Managing Lifecycle and Memory
- @StateObject should be used when a view is responsible for creating the
ObservableObject
. It ensures the object is only created once and survives view reloads. - @ObservedObject should be used when the
ObservableObject
is created elsewhere and passed into the view. This is ideal for scenarios where you don't want the view to manage the object's lifecycle. - @EnvironmentObject is perfect for global or app-wide shared state that many views need access to.