Opaque return types in Swift
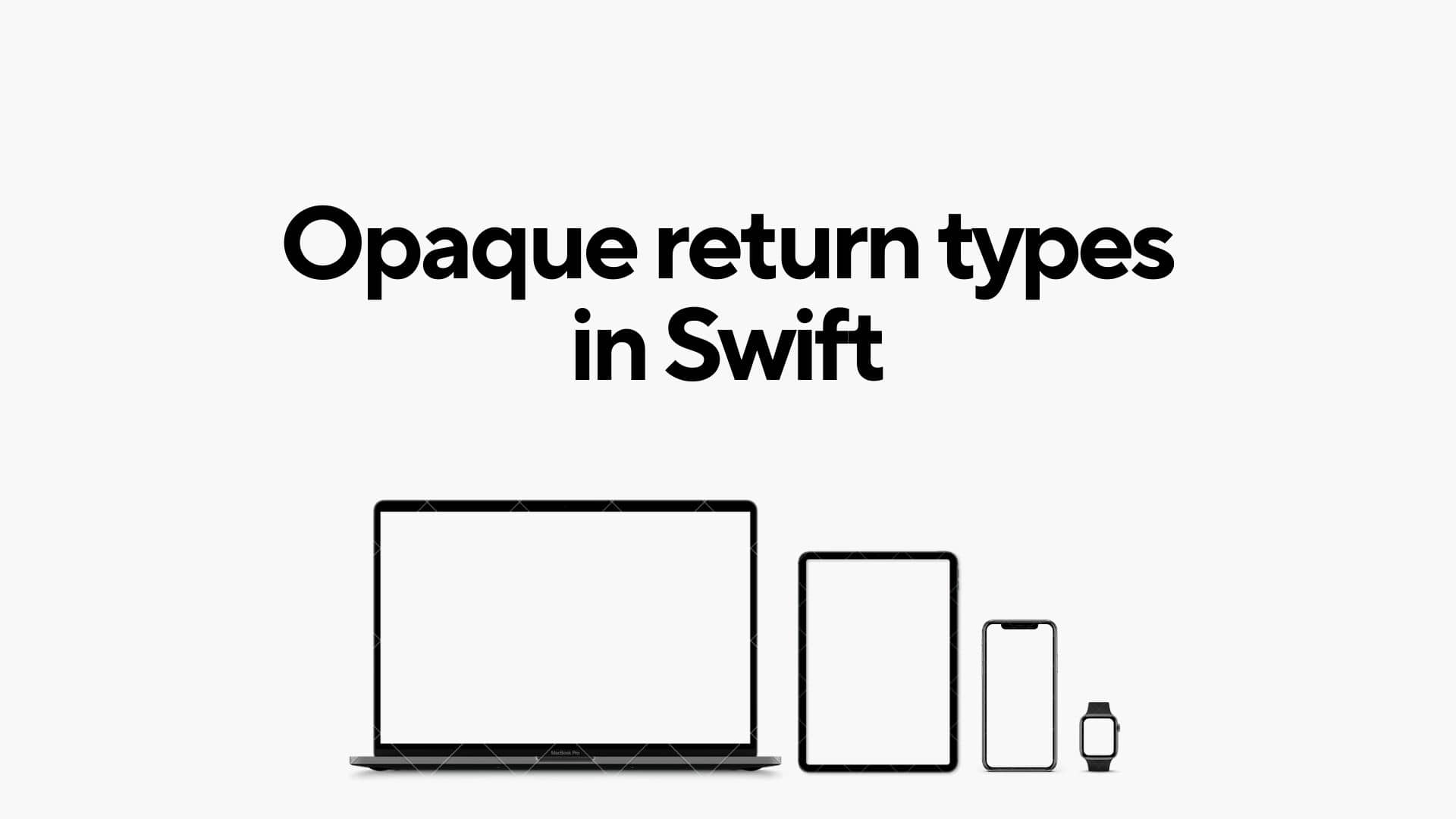
Opaque return types in Swift, introduced in Swift 5.1, provide a way to hide the concrete return type of a function while still preserving type safety. They are particularly useful when you want to hide implementation details while providing a clear and concise interface. Let's delve into opaque return types and their usage:
Definition:
An opaque return type is declared using the some
keyword followed by the protocol or protocol composition that the return type must conform to:
func makeSomeView() -> some View {
// Implementation details...
}
In this example, makeSomeView()
returns a type conforming to the View
protocol, but the concrete type is hidden from the caller.
Benefits:
- Abstraction: Opaque return types allow you to abstract away implementation details, focusing on what a function returns rather than how it's implemented.
- Type Safety: Opaque return types ensure type safety by guaranteeing that the returned value conforms to the specified protocol or composition.
Use Cases:
- Factory Methods: Use opaque return types to hide the implementation of factory methods, providing clients with an abstraction over the returned type.
- API Design: When designing APIs, use opaque return types to hide implementation changes and provide a stable interface to clients.
Limitations:
- Single Type: Opaque return types can only represent a single concrete type. If your function needs to return different types based on conditions, you may need to use generics or conditional logic.
- Limited Protocol Conformance: The protocol or composition used in the opaque return type must be explicitly defined. You cannot use opaque return types for existential types.
Example:
protocol Shape {
func draw() -> String
}
struct Circle: Shape {
func draw() -> String {
return "Drawing a circle"
}
}
struct Square: Shape {
func draw() -> String {
return "Drawing a square"
}
}
func randomShape() -> some Shape {
return Bool.random() ? Circle() : Square()
}
let shape1 = randomShape()
let shape2 = randomShape()
print(shape1.draw()) // Output varies based on randomness
print(shape2.draw()) // Output varies based on randomness
In this example, randomShape()
returns an opaque type conforming to the Shape
protocol. The concrete type is hidden, allowing the function to return either a Circle
or Square
instance based on randomness.
Conclusion:
Opaque return types in Swift provide a powerful abstraction mechanism, enabling you to hide implementation details and promote type safety in your code. By using opaque return types judiciously, you can design cleaner APIs and maintainable codebases while preserving flexibility and extensibility.