Operator Overloading in Swift
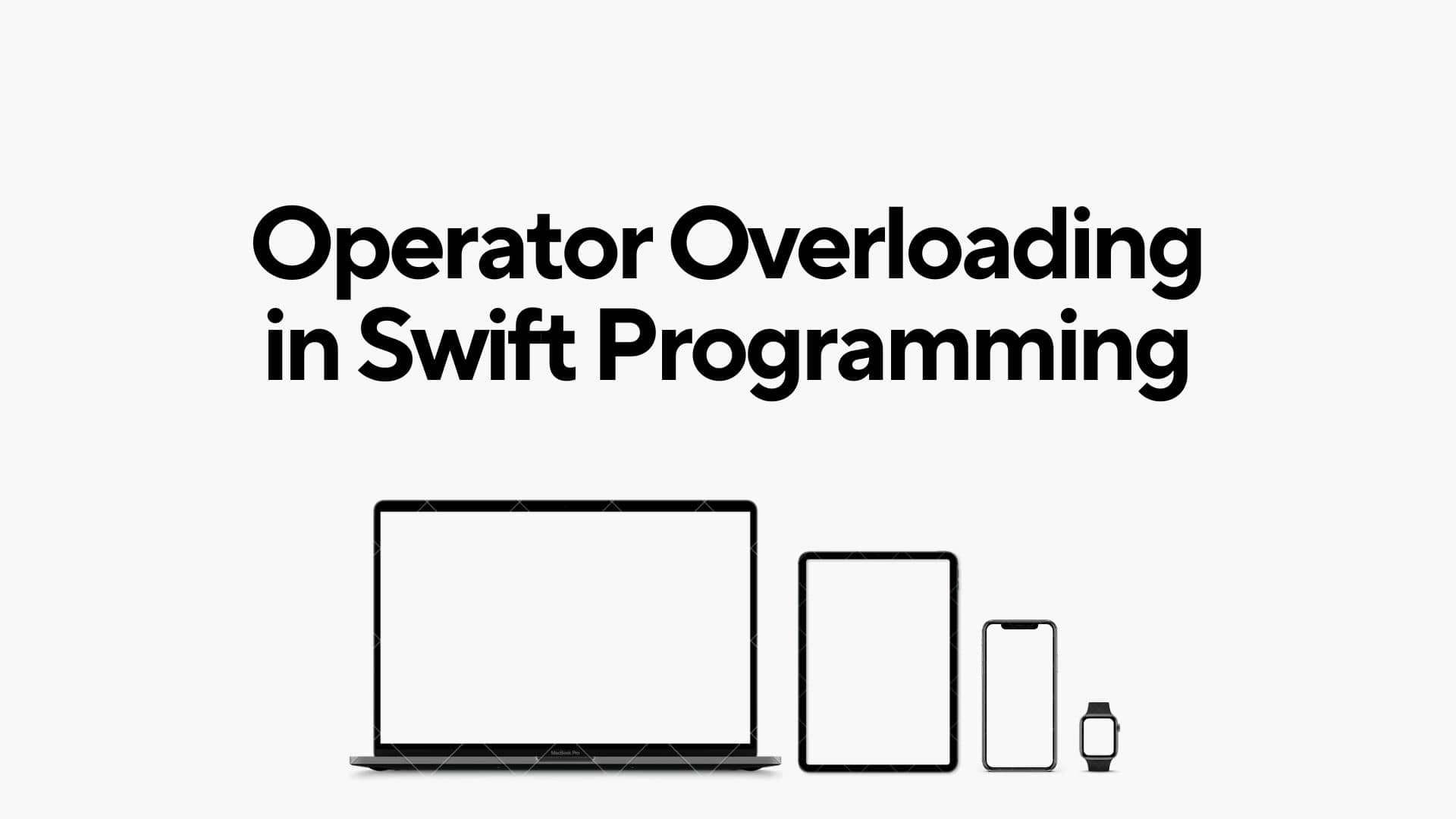
In Swift, operator overloading provides a powerful mechanism for extending the functionality of built-in operators to work with custom types. By defining custom implementations for operators like +
, -
, *
, and more, developers can imbue their own types with expressive and intuitive behavior, enhancing code readability and maintainability. In this guide, we'll explore the concept of operator overloading in Swift, understand its syntax and conventions, and delve into practical examples showcasing its versatility and utility.
Introduction to Operator Overloading
Operator overloading allows developers to redefine the behavior of existing operators for their custom types. This means that operators such as +
, -
, *
, and others can be used with user-defined classes and structures, enabling intuitive and concise syntax for performing operations specific to those types.
Syntax of Operator Overloading
In Swift, operator overloading is achieved by defining global functions with the operator
keyword followed by the operator to be overloaded. Let's look at a simple example of overloading the addition operator +
for a custom Vector
type:
struct Vector {
var x, y: Double
}
extension Vector {
static func + (left: Vector, right: Vector) -> Vector {
return Vector(x: left.x + right.x, y: left.y + right.y)
}
}
In this example, we define a custom Vector
structure and then extend it to provide a custom implementation of the addition operator +
. This implementation allows us to add two Vector
instances together using the familiar +
syntax.
Using Operator Overloading
Once operators are overloaded for custom types, they can be used just like built-in operators. Let's see how we can use the custom +
operator with our Vector
type:
let vector1 = Vector(x: 2.0, y: 3.0)
let vector2 = Vector(x: 1.0, y: 4.0)
let result = vector1 + vector2
print("Result: (\(result.x), \(result.y))") // Output: Result: (3.0, 7.0)
In this example, we create two Vector
instances and add them together using the overloaded +
operator, resulting in a new Vector
instance with the summed components.
Precedence and Associativity
When overloading operators, it's important to consider precedence and associativity to ensure that the behavior is consistent with other operators in Swift. Precedence determines the order in which operators are evaluated in an expression, while associativity determines the grouping of operators with the same precedence. Swift provides default precedence and associativity for many operators, but custom operators can specify their own precedence and associativity using operator declarations.
Practical Examples of Operator Overloading
Operator overloading can be used in a wide range of scenarios to enhance code readability and expressiveness. Some practical examples include:
- Overloading arithmetic operators for custom numeric types like matrices or complex numbers.
- Overloading comparison operators (
==
,!=
,<
,>
, etc.) for custom types to define custom equality or ordering criteria. - Overloading bitwise operators (
&
,|
,<<
,>>
, etc.) for custom bit manipulation operations.
Operator Overloading in Swift Programming
Operator overloading in Swift provides developers with a powerful tool for extending the functionality of built-in operators to work with custom types. By defining custom implementations for operators, developers can create more expressive, intuitive, and concise code, enhancing the readability and maintainability of their Swift codebases. Whether it's arithmetic operations, comparison operations, or bitwise operations, operator overloading allows Swift developers to unleash the full potential of their custom types. Happy coding!