Optionals ? vs Force Unwrap ! - SwiftUI
Understand the difference between ? and ! in SwiftUI with simple examples and tips for safer coding.
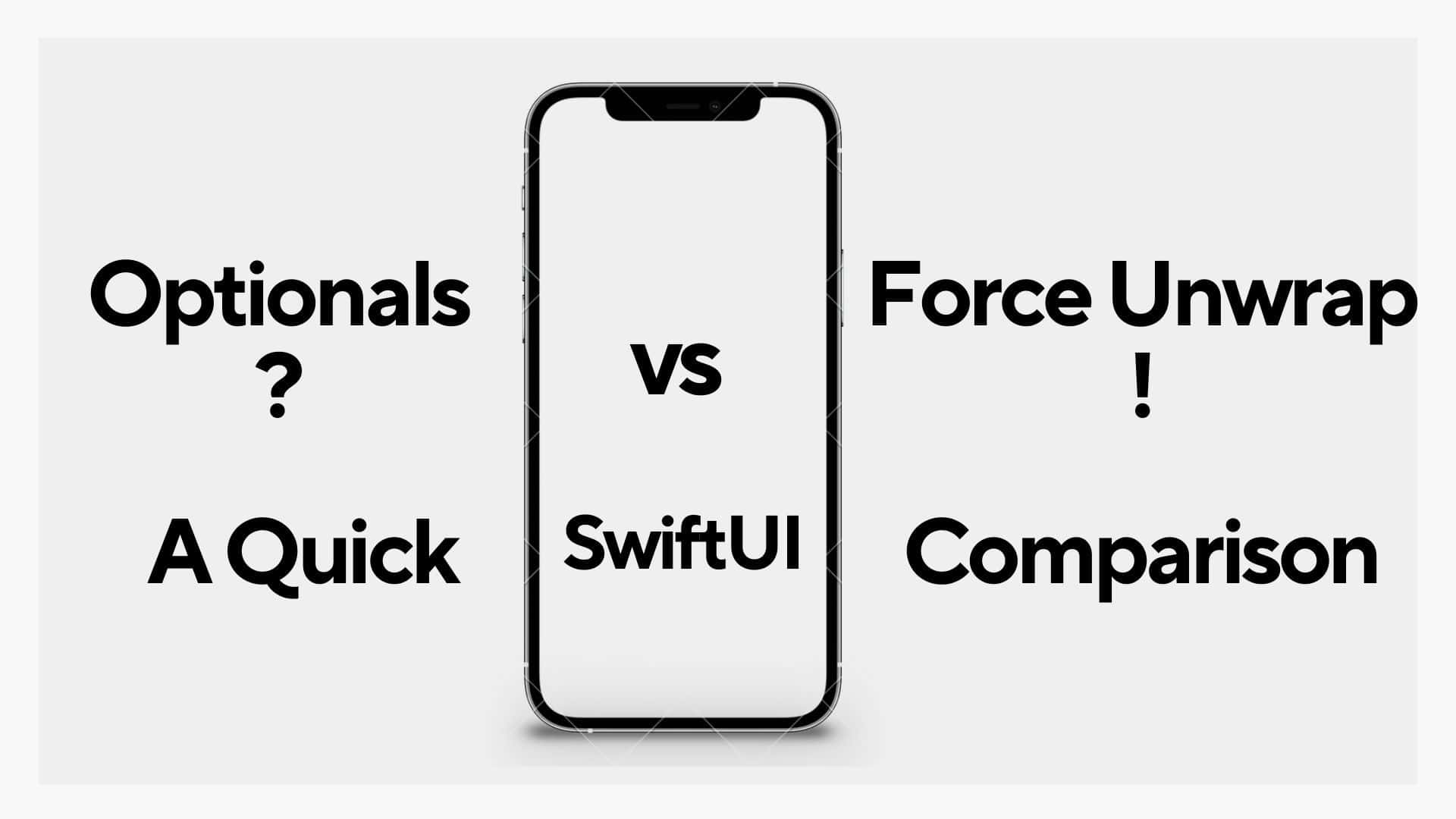
In Swift and SwiftUI, the question mark (?
) and exclamation mark (!
) are used to handle optional values, but they have very different meanings and use cases. Here's a detailed explanation to clarify their roles:
Optionals (?
)
An optional in Swift indicates that a value may or may not exist. It's a core feature for safely handling the absence of values.
Declaration
You declare a variable or constant as optional by appending a ?
to its type:
var name: String? // This variable can hold a String or nil
Key Behaviors
Initialization:
By default, an optional is nil
unless explicitly assigned a value.
var name: String?
print(name) // Output: nil
name = "Alice"
print(name) // Output: Optional("Alice")
Optional Binding:
Use if let
or guard let
to safely "unwrap" an optional and access its value.
if let unwrappedName = name {
print("Hello, \(unwrappedName)") // Safely accesses the value
} else {
print("Name is nil")
}
Optional Chaining:
You can call properties, methods, or subscripts on an optional, and it will return nil
if the optional is nil
.
let firstLetter = name?.first // Safely gets first letter if name is not nil
Default Values with Nil-Coalescing:
Provide a default value to use if the optional is nil
:
let displayName = name ?? "Guest"
Forced Unwrapping (!
)
The exclamation mark is used to forcefully unwrap an optional, assuming it contains a value. If the optional is nil
, it will trigger a runtime crash.
Use Case
- Forced Unwrapping:
Directly access the value of an optional:
var name: String? = "Alice"
print(name!) // Output: Alice
name
were nil
, this would crash your app with a fatal error: Unexpectedly found nil while unwrapping an Optional
.Implicitly Unwrapped Optionals:
If you are certain an optional will always have a value after it is initialized, you can declare it with !
:
var name: String! = "Alice"
print(name) // No need to unwrap explicitly
However, this is risky and should only be used in specific cases, such as dependency injection or interface builder outlets in UIKit.
When to Use ?
vs !
Use ?
:
- When a value might be nil, and you want to handle it safely.
- To ensure your app doesn't crash by checking for nil before unwrapping.
Use !
:
- When you are 100% certain an optional will have a value at the time of access.
- Typically used for outlets in
@IBOutlet
(e.g., SwiftUIUIViewRepresentable
).
Example in SwiftUI
Using ?
(Safe Handling)
struct ContentView: View {
@State private var name: String? = nil
var body: some View {
VStack {
Text(name ?? "Guest") // Safely use optional with a default value
Button("Set Name") {
name = "Alice"
}
}
}
}
Using !
(Risky but Concise)
struct ContentView: View {
@State private var name: String! = nil // Implicitly unwrapped optional
var body: some View {
VStack {
Text(name) // Assumes name is not nil; will crash otherwise
Button("Set Name") {
name = "Alice"
}
}
}
}
Best Practices
- Prefer
?
over!
: Always prioritize safe handling of optionals. Forced unwrapping is best avoided unless absolutely necessary. - Use
!
judiciously: Only use it when you are 100% sure the optional will never benil
at runtime.