Property Observers in Swift
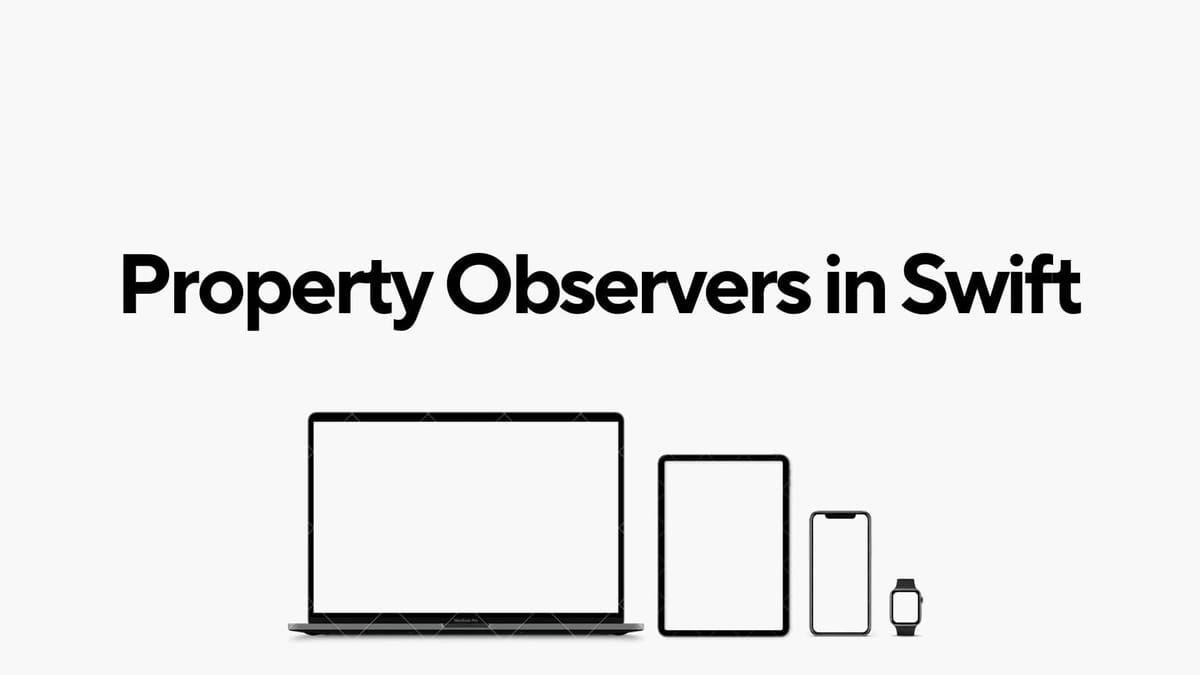
Property observers in Swift allow you to observe and respond to changes in property values. They are available for both stored properties and computed properties. There are two types of property observers:
- willSet: This observer is called just before the value of the property is set. It provides the new value as a constant parameter, which you can use to perform any necessary actions before the assignment takes place.
- didSet: This observer is called immediately after the value of the property is set. It provides the old value of the property as a constant parameter, allowing you to perform actions based on the previous value.
Here's an example demonstrating property observers:
class Temperature {
var celsius: Double {
willSet {
print("Setting new value: \(newValue) degrees Celsius")
}
didSet {
if celsius > oldValue {
print("Temperature increased from \(oldValue) to \(celsius) degrees Celsius")
} else if celsius < oldValue {
print("Temperature decreased from \(oldValue) to \(celsius) degrees Celsius")
} else {
print("Temperature remained the same at \(celsius) degrees Celsius")
}
}
}
init(celsius: Double) {
self.celsius = celsius
}
}
var currentTemperature = Temperature(celsius: 25.0)
// Setting new value: 30.0 degrees Celsius
// Temperature increased from 25.0 to 30.0 degrees Celsius
currentTemperature.celsius = 20.0
// Setting new value: 20.0 degrees Celsius
// Temperature decreased from 30.0 to 20.0 degrees Celsius
currentTemperature.celsius = 20.0
// Setting new value: 20.0 degrees Celsius
// Temperature remained the same at 20.0 degrees Celsius
In this example, we define a Temperature
class with a celsius
property. We use willSet
to print a message before setting the new value and didSet
to print a message after setting the new value, comparing it with the old value.
Property observers are useful for performing additional actions when a property's value changes, such as updating UI elements, logging, or maintaining consistency between related properties.
- Thread Safety: Property observers are always called on the same thread that the property's value is modified on. This ensures that property observer code is executed in a predictable manner without the need for additional synchronization mechanisms.
- Not Triggered by Initialization: Property observers are not called when a property is initialized during the declaration within a class or struct. They are only triggered when the property's value changes after initialization.
- Limited Use with Lazy Properties: Property observers cannot be used with lazy stored properties because lazy properties are initialized only once, and property observers are not called during initialization.
- Cannot Be Used with Constant Properties: Property observers cannot be used with constant (immutable) properties (
let
properties) because their values cannot change after initialization. - Order of Execution: If both
willSet
anddidSet
observers are specified for a property,willSet
is always called before the property's value changes, anddidSet
is always called after the change has occurred. This ensures a consistent order of execution. - Accessing Property within Observers: Inside property observers, you can access other properties and methods of the containing class or struct, including
self
. However, accessing the property being observed directly within the observer's definition can lead to infinite recursion and should be avoided. - Can Be Used with Computed Properties: Property observers can also be used with computed properties. In this case, they are triggered when the computed property's setter is called, even though the property itself doesn't store any value.
- Multiple Observers: You can define multiple
willSet
anddidSet
observers for a single property. They are executed in the order in which they are declared. - Subclassing Considerations: If a subclass overrides a property that has property observers in its superclass, the subclass can provide its own implementations of
willSet
anddidSet
observers. These observers are called instead of the superclass observers when the property is modified within the subclass.
Property observers provide a flexible mechanism for reacting to changes in property values, allowing you to customize the behavior of your classes and structs with precision.