Shorthand syntax in Swift
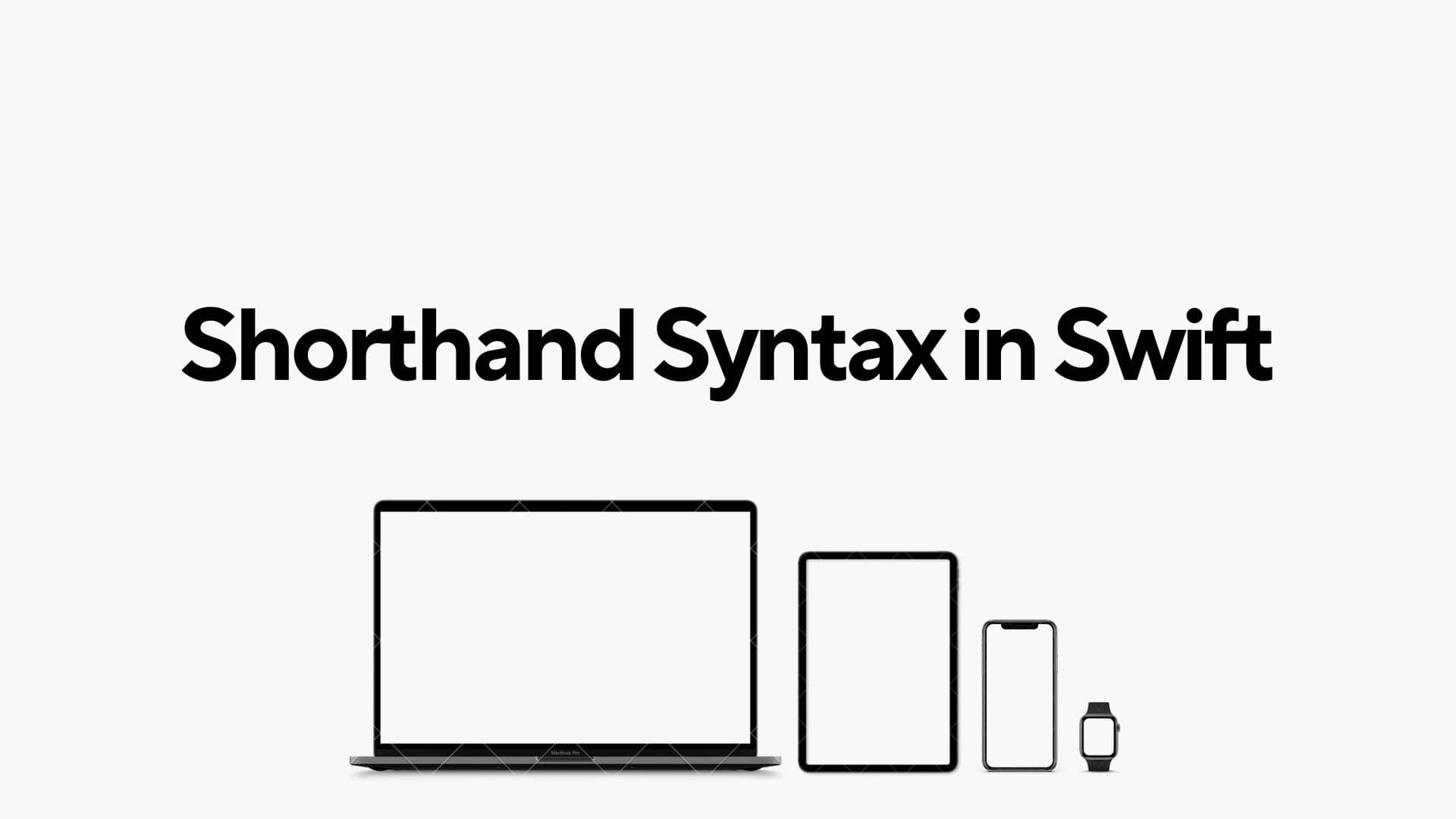
Shorthand syntax in Swift refers to concise and abbreviated ways of writing code that achieve the same functionality as longer, more verbose expressions. Swift provides several shorthand techniques to streamline common tasks and improve code readability. Let's explore some of the most commonly used shorthand syntax in Swift:
1. Type Inference:
Swift's type inference system allows you to omit explicit type declarations when the compiler can infer the type based on context. For example:
let number = 10 // Compiler infers number as Int
let text = "Hello" // Compiler infers text as String
2. Implicitly Unwrapped Optionals:
When you're sure that an optional will always have a value, you can declare it as implicitly unwrapped using the exclamation mark (!) at the end of the type declaration. This allows you to access the value without unwrapping it explicitly:
let optionalString: String! = "Hello"
print(optionalString) // No need for optional binding or forced unwrapping
3. Nil Coalescing Operator (??):
The nil coalescing operator provides a concise way to handle optionals by providing a default value if the optional is nil:
let name: String? = nil
let displayName = name ?? "Guest"
4. Optional Chaining (?.):
Optional chaining allows you to access properties, methods, and subscripts of optional values without unwrapping them explicitly. If any part of the chain is nil, the entire chain evaluates to nil:
let user: User? = User(name: "Alice", age: 30)
let userName = user?.name
5. Trailing Closures:
When a closure is the last argument of a function, you can use trailing closure syntax to move the closure outside of the parentheses, making the call site cleaner:
let numbers = [1, 2, 3, 4]
let sum = numbers.reduce(0) { $0 + $1 }
6. Shorthand Argument Names in Closures:
Swift allows you to use shorthand argument names (like $0, $1, etc.) in closures, which refer to the arguments of the closure in order:
let numbers = [1, 2, 3, 4]
let doubledNumbers = numbers.map { $0 * 2 }
7. Key-Value Coding (KVC) and Key-Value Observing (KVO) Shorthand:
In Swift, you can use key paths to access properties dynamically. Key paths provide a shorthand syntax for referring to properties by name:
let person = Person()
let name = person[keyPath: \.name]
8. Compact Map (flatMap) Operator:
The compact map operator (flatMap) allows you to simultaneously map and filter elements of a sequence, removing nil values:
let strings = ["1", "2", "three", "4"]
let numbers = strings.compactMap { Int($0) }
Shorthand syntax in Swift plays a crucial role in improving code conciseness, readability, and maintainability. By leveraging these shorthand techniques, Swift developers can write cleaner, more expressive code that is easier to understand and maintain. Whether it's type inference, optional chaining, or trailing closures, mastering shorthand syntax empowers developers to write more efficient and elegant Swift code.