Struct data type in Swift
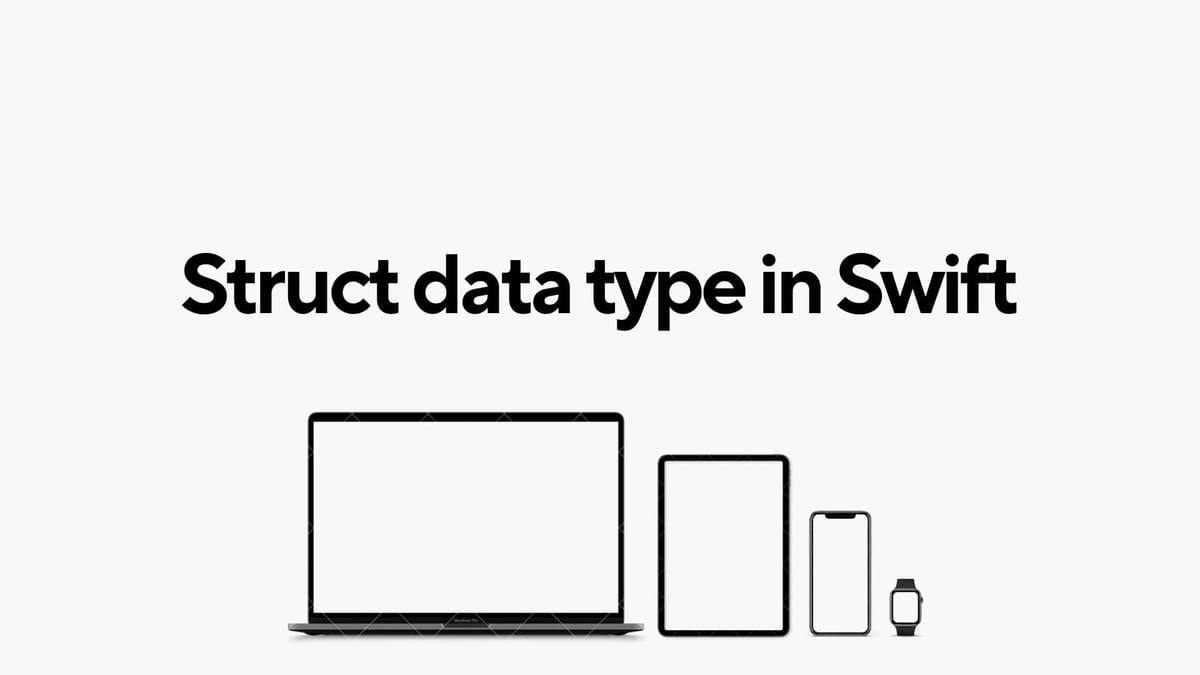
In Swift, a struct
is a value type that allows you to encapsulate related properties and behaviors into a single compound type. Structs are used to create custom data types and are similar to classes in many ways, but they have some key differences.
Here's a basic example of a struct in Swift:
struct Person {
var name: String
var age: Int
}
In this example, Person
is a struct with two properties: name
and age
. Each property has a type (String
and Int
, respectively).
You can create an instance of this struct and access its properties like this:
var person1 = Person(name: "John", age: 30)
print("Name: \(person1.name), Age: \(person1.age)") // Output: Name: John, Age: 30
You can also define methods within a struct:
struct Person {
var name: String
var age: Int
func sayHello() {
print("Hello, my name is \(name) and I'm \(age) years old.")
}
}
var person1 = Person(name: "John", age: 30)
person1.sayHello() // Output: Hello, my name is John and I'm 30 years old.
Some key points about structs in Swift:
- Value Semantics: Unlike classes, which are reference types, structs are value types. This means when you assign a struct to a new constant or variable, a copy of the struct is created, and modifications to one instance of a struct do not affect other instances.
- Immutability: You can mark instances of a struct as
let
to make them immutable (constant). This ensures that their properties cannot be changed after initialization. - Memberwise Initializers: Swift automatically generates memberwise initializers for structs, which allow you to create instances of the struct by providing values for each of its properties.
- Inheritance: Structs do not support inheritance, unlike classes.
Structs are commonly used to represent simple data structures, such as geometric shapes, coordinates, and other lightweight data types. They are preferred over classes in many cases due to their value semantics and performance benefits.
Here are some additional differences and features of structs in Swift compared to Classes:
- No Inheritance: Unlike classes, structs do not support inheritance. They cannot inherit properties or behaviors from other types, nor can they be subclassed. This can simplify code and prevent potential complexity.
- Value Semantics: As mentioned before, structs are value types, meaning they are copied when passed around in your code. This can lead to different behavior compared to classes, especially in scenarios where you expect copies of values rather than references to shared data.
- Copy-on-Write (COW): Swift employs copy-on-write optimization for structs. This means that the system only makes copies of a struct's data when necessary, such as when modifying a value that is shared with other instances. This optimization helps reduce unnecessary copying, improving performance.
- Mutability Control: While you can mark individual properties of a struct as
var
(mutable) orlet
(immutable), you cannot mark the entire struct itself asmutating
like you would with methods in classes. Struct methods that modify properties must be explicitly marked with themutating
keyword. - Automatic Synthesis of Member wise Initializers: Swift automatically generates member wise initializers for structs, which allow you to initialize instances by specifying values for each property. This can save you from writing boilerplate code for initializing structs.
- No De-initializers: Unlike classes, structs do not have deinitializers. They are deallocated as soon as they are no longer in use. This is because structs do not support inheritance or reference counting, so there is no need for cleanup logic when they are deallocated.
- Value and Reference Type Distinction: Swift makes a clear distinction between value types (like structs and enums) and reference types (like classes). This distinction is important for understanding how data is stored, passed around, and mutated in your code, which can help you write more predictable and efficient programs.
- Default Member wise Initializers: Unlike classes, structs get a default member wise initializer even if you define your own custom initializer. This behavior can be convenient but also means you need to be mindful of how your initializers interact with each other, especially when defining custom initialization logic.
Overall, understanding these differences can help you decide when to use structs versus classes in your Swift code and how to leverage their unique features effectively.