Swift functions to query Collections
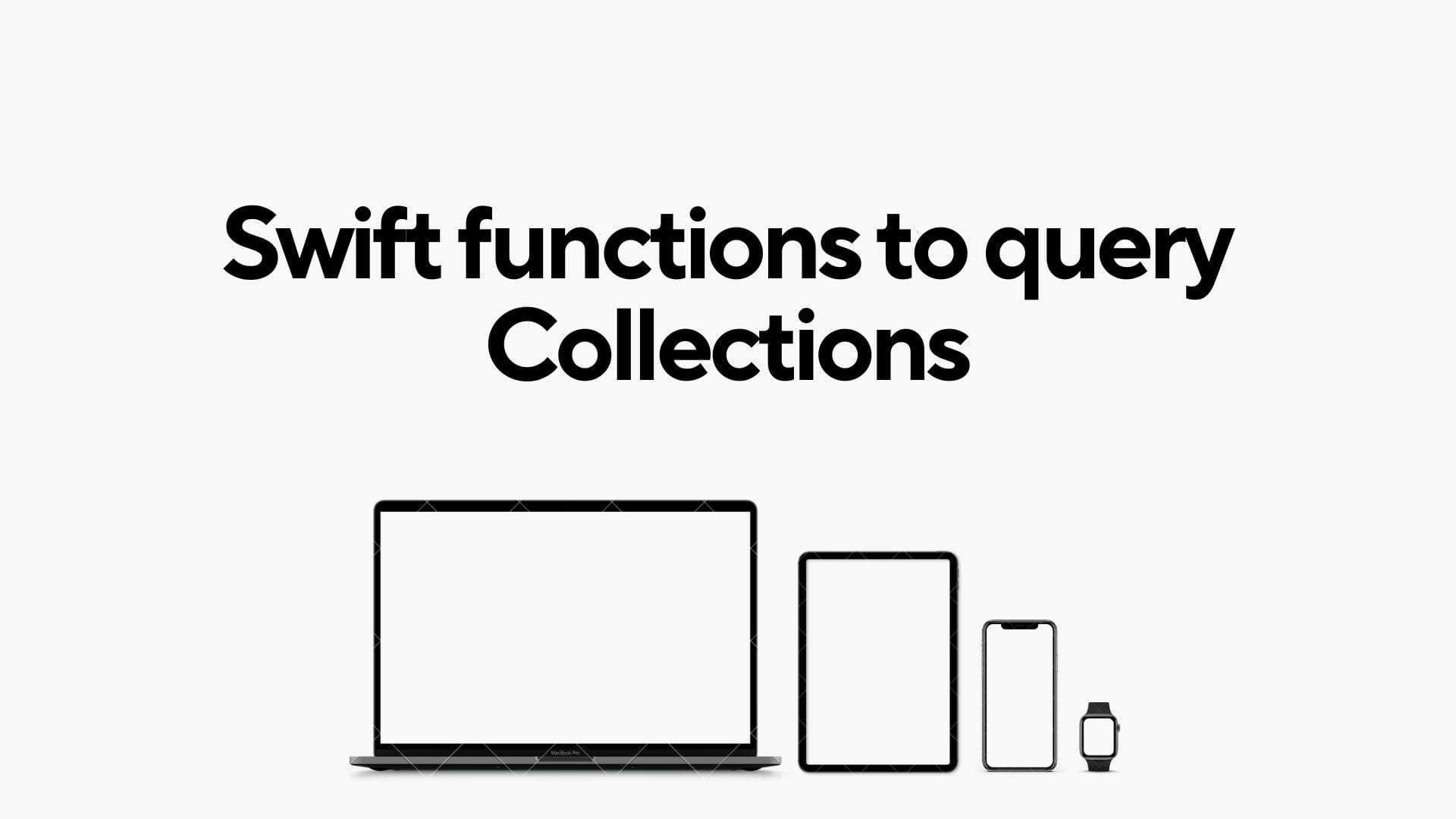
Swift functions are indeed powerful tools for working with collections, allowing for efficient and expressive manipulation of data. Whether you need to toggle a boolean, remove elements, check for containment, sort, reverse, or determine the count of elements, these functions offer concise and readable ways to achieve these operations. Swift's emphasis on clarity and safety is evident in the design of these functions, making code more maintainable and less error-prone.
Count: The count property is used to get the number of elements in a collection.
Code:
let numbers = [1, 2, 3, 4, 5]
let countOfNumbers = numbers.count
print(countOfNumbers)
Outputs: 5
toggle(): The toggle() function is used to toggle a boolean value. If the boolean variable is true
, it becomes false
, and if it's false
, it becomes true
.
Code:
var isOn = true
isOn.toggle()
print(isOn)
Outputs: false
remove(at:): The remove(at:) function is used to remove the element at a specific index from a mutable collection (like an array).
Code:
var numbers = [1, 2, 3, 4, 5]
numbers.remove(at: 2)
print(numbers)
Outputs: [1,2,4,5]
removeAll(): The removeAll() function is used to remove all elements from a mutable collection.
Code::
var numbers = [1, 2, 3, 4, 5]
numbers.removeAll()
print(numbers)
Outputs: []
contains(_:): The contains() function is used to check if a sequence (like an array) contains a specific element. It returns a boolean value indicating whether the element is present or not. Example:
let numbers = [1, 2, 3, 4, 5]
let containsThree = numbers.contains(3)
print(containsThree)
Outputs: true
sorted(): The sorted() function is used to return a new array with the elements of the original array sorted in ascending order. Example:
let unsortedNumbers = [5, 2, 8, 1, 3]
let sortedNumbers = unsortedNumbers.sorted()
print(sortedNumbers)
Outputs: [1, 2, 3, 5, 8]
reversed(): The reversed() is used to return a new array with the elements of the original array in reverse order. Example:
let numbers = [1, 2, 3, 4, 5]
let reversedNumbers = numbers.reversed()
print(Array(reversedNumbers))
Outputs: [5, 4, 3, 2, 1]
map(_:): The map() function is used to transform each element of a collection using a provided closure and return a new collection with the transformed values. Example:
let numbers = [1, 2, 3, 4, 5]
let squaredNumbers = numbers.map { $0 * $0 }
print(squaredNumbers)
Outputs: [1, 4, 9, 16, 25]
reduce(_:_:): The reduce() function is used to combine all elements of a collection into a single value. It takes an initial value and a closure that specifies how to combine the elements. Example:
let numbers = [1, 2, 3, 4, 5]
let sum = numbers.reduce(0) { $0 + $1 }
print(sum)
Outputs: 15
filter(_:): The filter() function is used to create a new collection containing only the elements that satisfy a given condition specified by a closure. Example:
let numbers = [1, 2, 3, 4, 5]
let evenNumbers = numbers.filter { $0 % 2 == 0 }
print(evenNumbers)
Outputs: [2, 4]
isEmpty: The isEmpty property returns a boolean value indicating whether the collection is empty or not. It's particularly useful for conditionally checking if you need to perform certain operations on a collection depending on whether it contains elements.
Code:
let numbers = [1, 2, 3, 4, 5]
let isNumbersEmpty = numbers.isEmpty
print(isNumbersEmpty)
let emptyArray: [Int] = []
let isEmptyArrayEmpty = emptyArray.isEmpty
print(isEmptyArrayEmpty)
Outputs: false
Outputs: true
These querying functions provide a powerful set of tools for manipulating and transforming collections in a concise and expressive manner in Swift. They contribute to Swift's functional programming paradigm, allowing developers to write code that is both readable and efficient.