Swift iOS vs Kotlin Android: A Quick Comparison
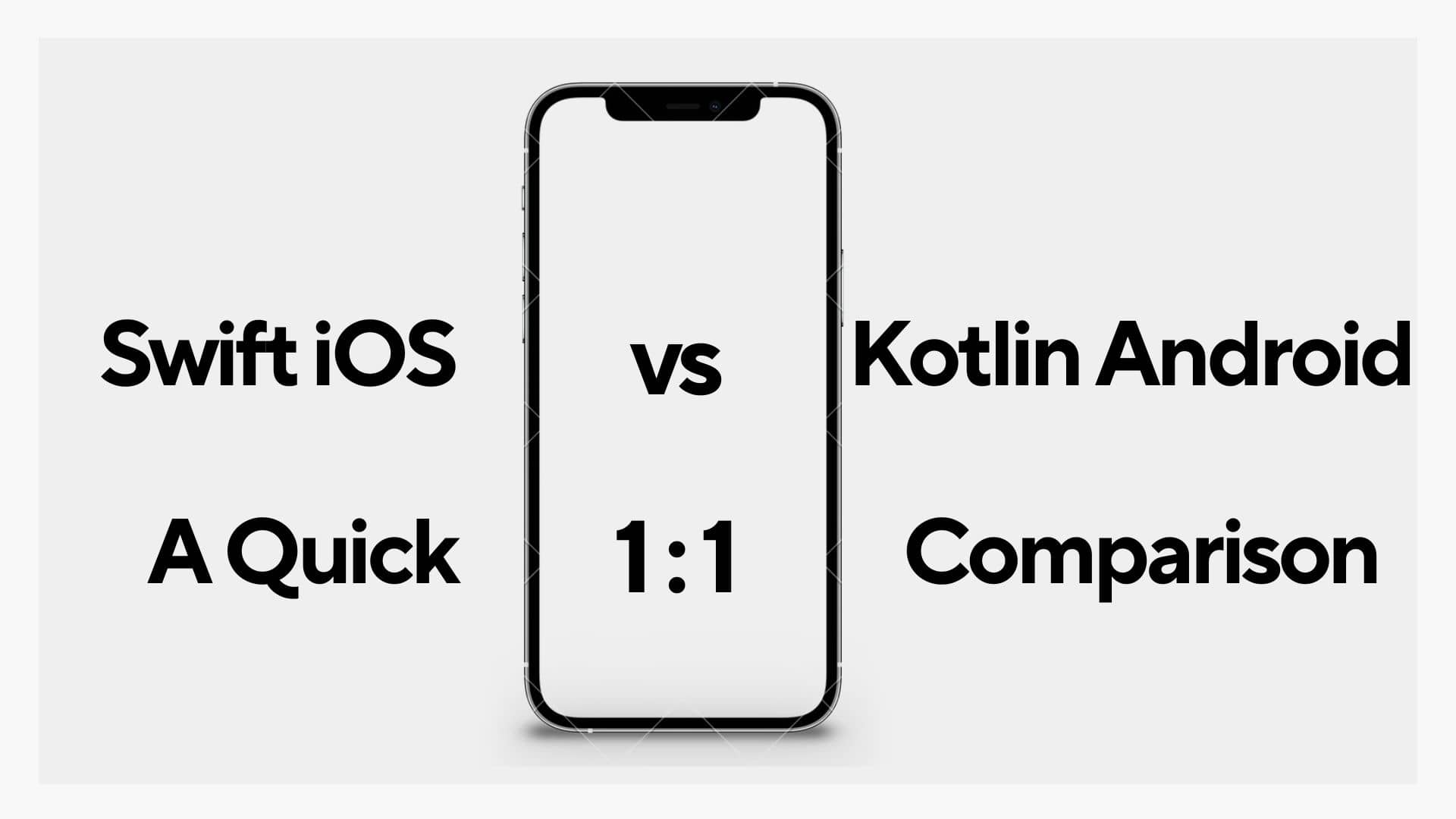
Mobile app development has become a dynamic and competitive field, with two major players dominating the market: iOS and Android. Swift and Kotlin are the respective programming languages for iOS and Android development, each boasting unique features and capabilities.
For developers looking to create applications for these platforms, the choice of programming language is crucial.
In this article, we'll explore into a detailed comparison of Swift, the language used for iOS development, and Kotlin, the language of choice for Android development.
1. Overview of Swift and Kotlin:
Swift: Developed by Apple in 2014.
Designed for iOS, macOS, watchOS, and tvOS.
Known for its simplicity, readability, and performance optimization.
Kotlin: Introduced by JetBrains in 2011.
Officially supported by Google for Android development since 2017.
Built on the Java Virtual Machine (JVM), making it interoperable with Java.
2. Syntax and Readability:
Swift: Influenced by languages like Objective-C, Python, and Ruby.
Features a concise and expressive syntax, reducing boilerplate code.
Emphasizes code readability and simplicity.
Here's a simple Swift code snippet for a basic "Hello, World!" application:
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let greeting = "Hello, World!"
print(greeting)
}
}
Kotlin: Designed by JetBrains, adopted by Google for Android development. Kotlin's syntax is concise, and it introduces various features that enhance developer productivity.
Here's the equivalent "Hello, World!" code snippet in Kotlin:
fun main() {
val greeting = "Hello, World!"
println(greeting)
}
Both languages offer simplicity and readability, but the choice between them often depends on personal preference and the specific requirements of the project.
3. Concurrency and Multithreading:
Swift: Leverages Grand Central Dispatch (GCD) for concurrent programming.
Provides async/await support, simplifying asynchronous code.
Offers thread safety through features like thread-safe collections.
Kotlin: Utilizes coroutines for asynchronous programming.
Offers structured concurrency for better control over parallel tasks.
Simplifies thread management with coroutine support, reducing callback hell.
4. Platform Integration:
Swift: Seamlessly integrates with Apple's ecosystem, including hardware features. Supports native iOS frameworks like Core Data, Core Animation, and UIKit. Exclusive to Apple platforms, limiting cross-platform development.
Here's an example using Core Data to save and fetch data:
import CoreData
// Code for saving and fetching data using Core Data in Swift
// ...
Kotlin: Offers excellent integration with existing Java libraries and frameworks. Capitalizes on the Android SDK and easily interfaces with Java code.
Supports cross-platform development through tools like Kotlin/Native.
Here's an example using Room to define and interact with a SQLite database:
import androidx.room.Entity
import androidx.room.PrimaryKey
@Entity(tableName = "user")
data class User(
@PrimaryKey(autoGenerate = true)
val id: Int = 0,
val name: String,
val age: Int
)
// Code for interacting with the Room database in Kotlin
// ...
5. Community and Support:
Swift: Thriving community of iOS developers with active support from Apple.
Regular updates and improvements through Swift Evolution process.
Extensive documentation and a rich ecosystem of third-party libraries.
Kotlin: Growing community with support from JetBrains and Google.
Regular updates and improvements based on community feedback.
Comprehensive documentation and compatibility with existing Java resources.
6. Learning Curve:
Swift: Designed to be beginner-friendly, especially for those transitioning from other languages.
Features Playground, an interactive learning environment.
Requires developers to adapt to Apple's specific design patterns.
Kotlin: Smooth learning curve for Java developers due to interoperability.
Offers concise syntax and modern language features for enhanced productivity.
Suitable for developers familiar with Java or other JVM languages.
7. Performance:
Swift: Highly optimized performance, particularly in terms of speed and memory management.
Native compilation contributes to efficient execution on Apple hardware.
Kotlin: Excellent performance, comparable to Java, thanks to JVM optimization.
Compiles to bytecode, allowing efficient execution on the Android runtime (ART).
Conclusion:
Choosing between Swift and Kotlin ultimately depends on the platform, project requirements, and personal preferences. Swift excels in the iOS ecosystem, providing seamless integration with Apple's hardware and software. On the other hand, Kotlin offers a versatile option for Android development, with the added advantage of cross-platform compatibility. Both languages have their strengths and are continually evolving, ensuring a vibrant landscape for mobile app development on iOS and Android platforms.