Swift Labeled Statements
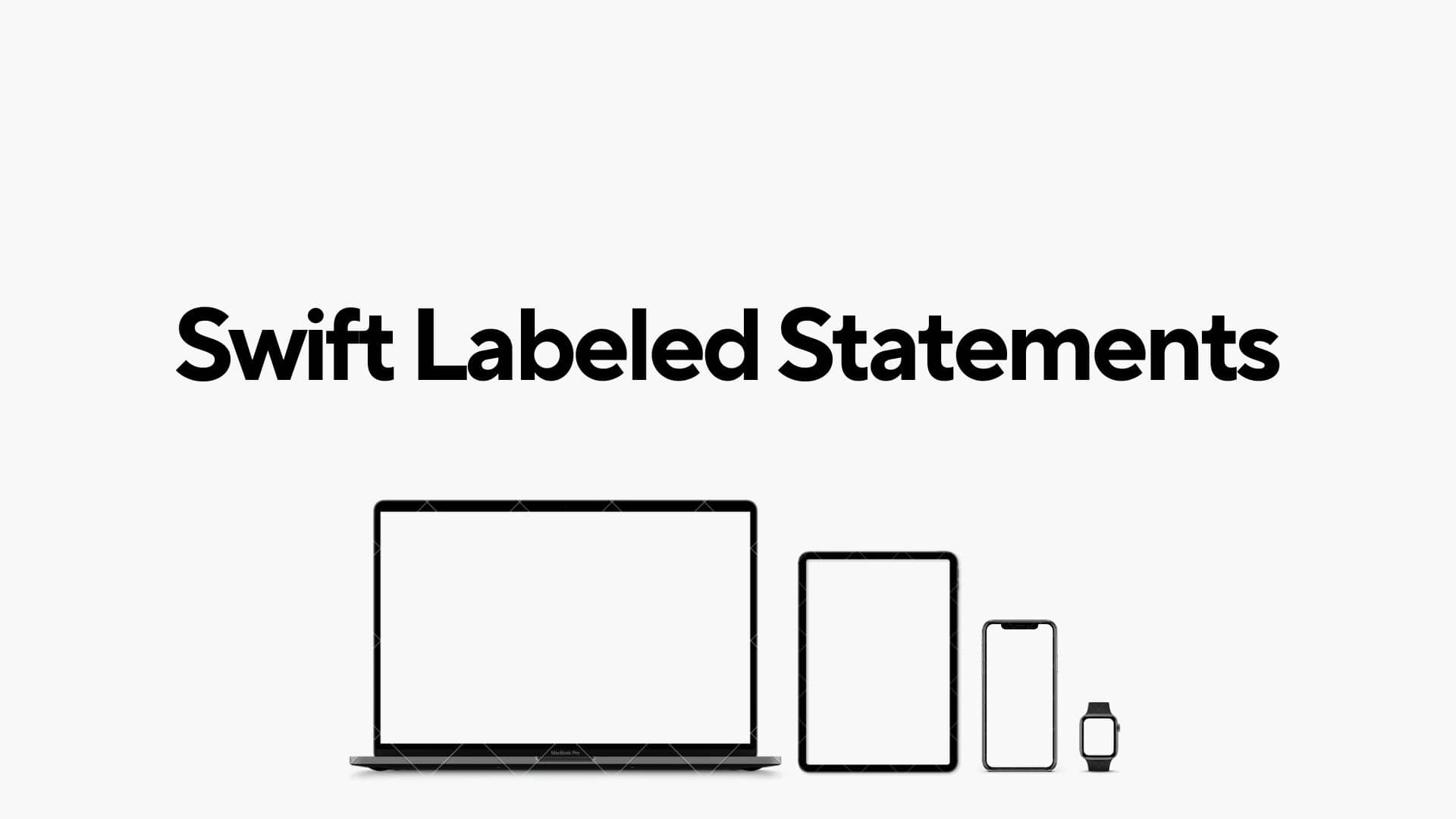
In the world of Swift programming, labeled statements offer a unique way to manipulate control flow, providing developers with greater clarity and flexibility in managing complex logic. While labeled statements might not be as commonly used as other control flow constructs, such as loops and conditionals, they can be invaluable in certain scenarios where precise control is necessary. In this guide, we'll explore the concept of labeled statements in Swift, understand their syntax and usage, and delve into practical examples where they shine.
Introduction to Labeled Statements
Labeled statements in Swift allow developers to assign custom identifiers to loops (such as for
, while
, repeat-while
) and conditional statements (if
, switch
). These labels provide a unique identifier for the statement, enabling more precise control over nested loops and conditionals.
Syntax of Labeled Statements
The syntax for creating a labeled statement is straightforward:
labelName: statement
Here, labelName
is the custom identifier assigned to the statement. It's followed by a colon (:
) to indicate the label, and then the actual statement. Let's explore how labeled statements can be used with different control flow constructs.
Using Labeled Statements with Loops
Labeled statements are particularly useful in nested loop scenarios, where you need to break out of multiple levels of looping. Consider the following example:
outerLoop: for i in 1...3 {
for j in 1...3 {
if i * j == 6 {
print("Breaking at i = \(i), j = \(j)")
break outerLoop
}
print("i = \(i), j = \(j)")
}
}
In this example, outerLoop
is a label assigned to the outer for
loop. When the condition i * j == 6
is met, the break
statement with outerLoop
label is executed, causing both loops to terminate.
Labeled Statements with Conditional Statements
Similarly, you can use labeled statements with conditional statements to control the flow of execution. Consider the following example using if
statements:
outerIf: if condition1 {
if condition2 {
print("Both conditions met")
break outerIf
}
print("Only condition1 met")
} else {
print("Condition1 not met")
}
In this scenario, outerIf
acts as a label for the outer if
statement. When condition2
is met, the break outerIf
statement causes the execution to jump to the end of the outerIf
block.
Practical Examples of Labeled Statements
Let's explore a practical example where labeled statements can enhance code readability and control flow. Suppose we have a nested loop structure where we want to find a specific value and break out of both loops when it's found:
let targetValue = 10
var found = false
searchLoop: for i in 1...5 {
for j in 1...5 {
if i * j == targetValue {
found = true
break searchLoop
}
}
}
if found {
print("Target value found!")
} else {
print("Target value not found")
}
In this example, the searchLoop
label allows us to break out of both loops once the target value is found, improving code readability and reducing complexity.
Labeled Statements in Swift
Labeled statements in Swift provide developers with a powerful tool for managing control flow in complex scenarios. By assigning custom identifiers to loops and conditional statements, labeled statements enhance code clarity and maintainability, particularly in situations involving nested control flow structures. While labeled statements should be used judiciously to avoid code clutter, they offer a valuable mechanism for precise control over program execution.
As you continue your Swift journey, consider incorporating labeled statements into your coding arsenal, especially in scenarios where nested control flow needs to be managed with precision. With a clear understanding of labeled statements, you'll be well-equipped to write more expressive and maintainable Swift code. Happy coding!