Tuple Pattern Matching in Swift
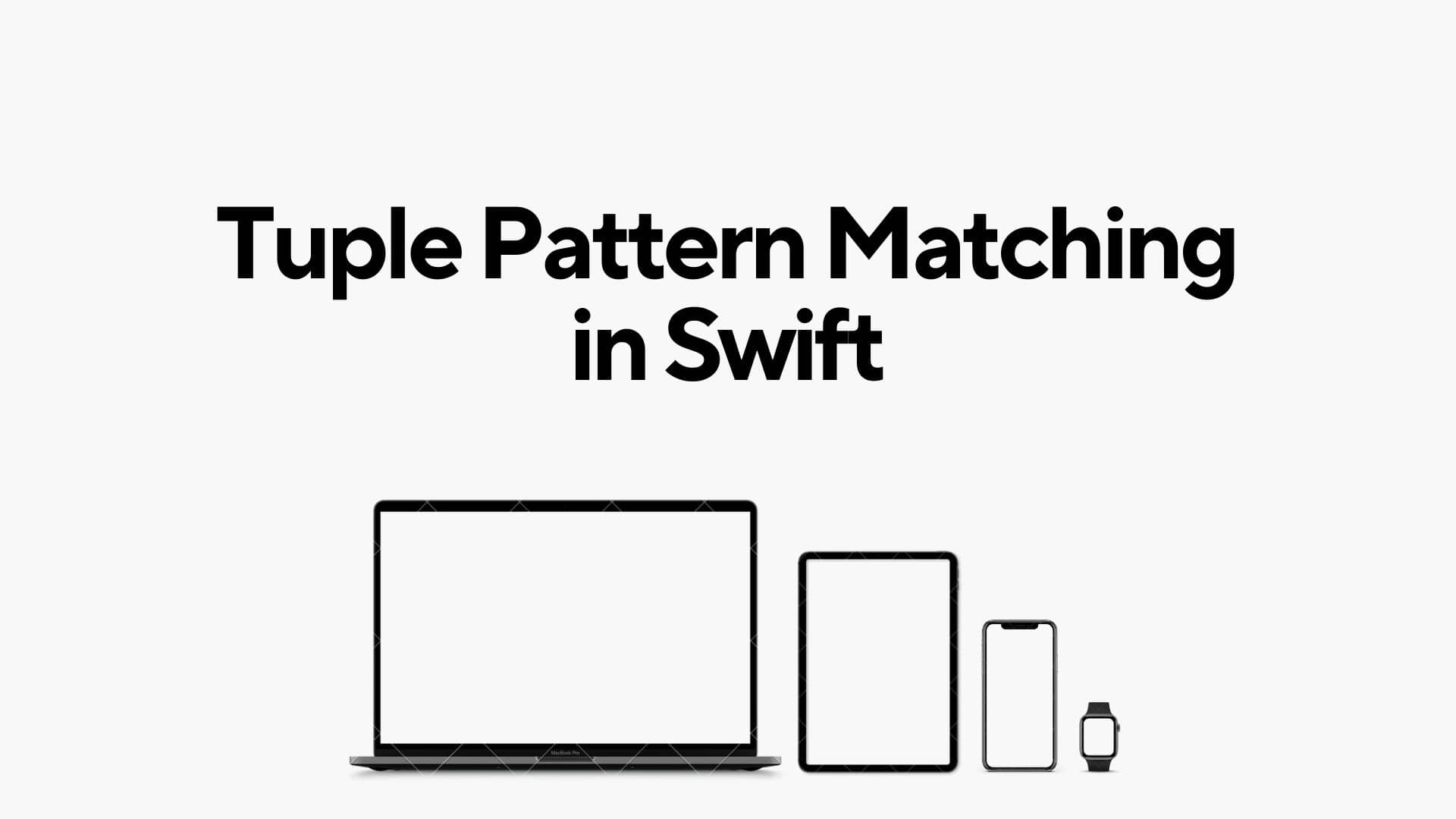
In the realm of Swift programming, tuple pattern matching offers a powerful mechanism for extracting and working with structured data. Tuples allow developers to group multiple values together into a single compound value, and pattern matching enables precise and elegant extraction of these values based on predefined patterns. In this comprehensive guide, we'll explore the concept of tuple pattern matching in Swift, understand its syntax and capabilities, and delve into practical examples where it shines.
Introduction to Tuple Pattern Matching
Tuple pattern matching is a feature in Swift that enables developers to destructure tuples and extract individual elements based on a specified pattern. This pattern can include variable names, wildcards, and even nested patterns, providing flexibility and expressiveness in handling complex data structures.
Syntax of Tuple Pattern Matching
The syntax for tuple pattern matching involves specifying the pattern within a case
statement, typically within a switch
statement or as part of an assignment. Let's explore the basic syntax:
let tuple = (value1, value2, value3)
switch tuple {
case (let x, let y, let z):
// Access individual values using x, y, and z
print("Values: \(x), \(y), \(z)")
default:
// Default case
break
}
In this example, the switch
statement matches the tuple against the pattern (let x, let y, let z)
, where let x
, let y
, and let z
are variable bindings that capture the individual elements of the tuple.
Variable Bindings and Wildcards
Tuple pattern matching supports variable bindings, allowing developers to extract values and bind them to named variables. Additionally, wildcards (_
) can be used to ignore specific elements of the tuple. Consider the following example:
let point = (3, 5)
switch point {
case (let x, _):
// Extract the x-coordinate and ignore the y-coordinate
print("x-coordinate: \(x)")
default:
break
}
Here, let x
captures the first element of the tuple (3
), while the wildcard _
ignores the second element.
Nested Patterns and Value Matching
Tuple pattern matching supports nested patterns, enabling developers to match nested tuples or other patterns within tuples. This feature provides a powerful mechanism for handling complex data structures. Let's explore a practical example:
let person = ("John", (30, "Developer"))
switch person {
case let (name, (age, occupation)):
// Extract name, age, and occupation from the nested tuple
print("Name: \(name), Age: \(age), Occupation: \(occupation)")
default:
break
}
In this example, we match the outer tuple against the pattern let (name, (age, occupation))
, extracting the name, age, and occupation from the nested tuple.
Practical Examples of Tuple Pattern Matching
Tuple pattern matching is particularly useful in scenarios involving functions that return tuples, such as parsing JSON data or extracting data from APIs. Let's consider an example where we parse a JSON response containing user information:
let json = """
{
"name": "Alice",
"age": 25,
"email": "alice@example.com"
}
"""
if let data = json.data(using: .utf8),
let jsonObject = try? JSONSerialization.jsonObject(with: data, options: []) as? [String: Any],
let (name, age, email) = (jsonObject["name"], jsonObject["age"], jsonObject["email"]) as? (String, Int, String) {
print("Name: \(name), Age: \(age), Email: \(email)")
} else {
print("Failed to parse JSON")
}
In this example, we parse the JSON response and extract the name, age, and email using tuple pattern matching within a conditional binding.
Tuple Pattern Matching for Structured Data Handling
Tuple pattern matching in Swift provides a powerful mechanism for working with structured data, allowing developers to extract and manipulate individual elements of tuples with precision and elegance. Whether it's parsing JSON responses, handling function return values, or deconstructing complex data structures, tuple pattern matching offers a versatile tool for Swift developers.
As you continue your Swift journey, consider incorporating tuple pattern matching into your coding arsenal. Experiment with different patterns, explore nested patterns, and leverage variable bindings and wildcards to handle diverse data scenarios. With a clear understanding of tuple pattern matching, you'll be well-equipped to tackle complex data handling tasks and write more expressive and maintainable Swift code. Happy coding!