UIKit Framework for crafting iOS User Interfaces
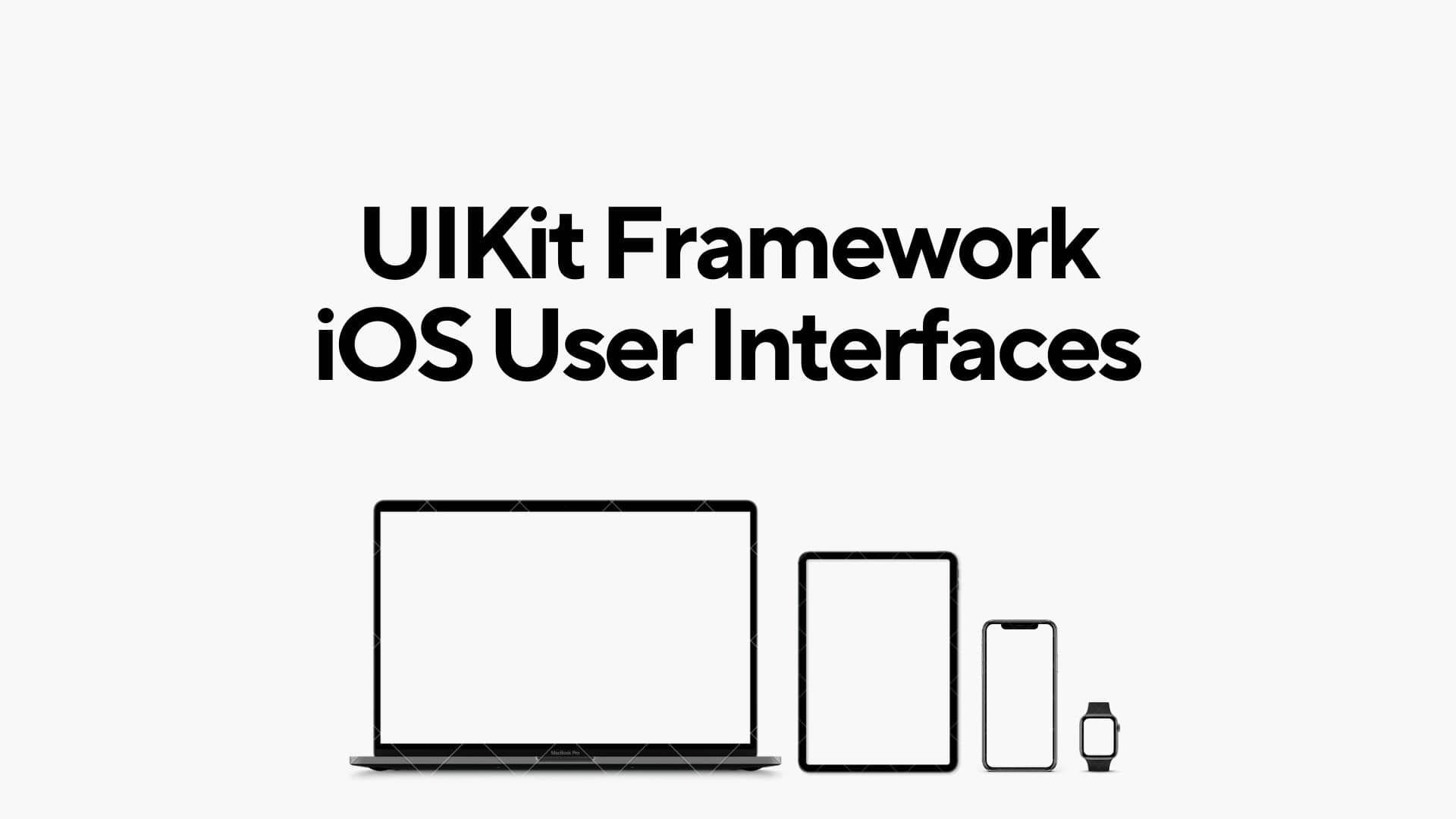
In the ever-evolving landscape of iOS app development, UIKit stands as the cornerstone framework for crafting intuitive and visually appealing user interfaces. If you're a tech enthusiast eager to embark on the captivating journey of iOS app development, understanding UIKit is not just a skill—it's a gateway to bringing your creative visions to life. In this comprehensive tutorial, we'll delve into the UIKit framework, unraveling its intricacies and guiding you through the process of building captivating user interfaces for your iOS applications.
Introduction to UIKit: The Heartbeat of iOS User Interfaces
At the heart of iOS lies UIKit, a framework that encapsulates the fundamental building blocks for creating user interfaces. From buttons and labels to intricate views and navigation controllers, UIKit provides the tools needed to breathe life into the visual aspects of your iOS applications. Let's embark on a journey to understand the key components of UIKit and how they come together to shape the user experience.
The Building Blocks: Views and View Controllers
UIKit revolves around the concept of views and view controllers. A view represents a rectangular area on the screen, while a view controller manages the content displayed within that area. Understanding this duo is crucial for crafting modular and scalable user interfaces.
- Views: The Visual Elements
Views are the visual elements that users interact with—buttons, labels, images, and more. Each view is an instance of theUIView
class, and UIKit provides a rich set of predefined views that you can leverage.
let myButton = UIButton(type: .system)
myButton.setTitle("Tap me!", for: .normal)
Here, we create a button using UIButton
, setting its title for the normal state. The .system
type ensures that the button follows the system's default appearance.
- View Controllers: Orchestrating the Show
View controllers manage the presentation and behavior of views. They act as intermediaries between the app's data and the views displayed on the screen. TheUIViewController
class forms the foundation for creating view controllers.
class MyViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Your setup code goes here
}
}
In this example, MyViewController
is a custom view controller where you can initialize and configure your views.
Storyboard: A Visual Canvas for UI Design
Enter the world of Interface Builder and storyboards—an integral part of UIKit that allows you to design your user interface visually. Storyboards provide a visual representation of your app's screens and the flow between them. Here's a glimpse of how you can leverage storyboards:
- Creating a New Storyboard:
Start by creating a new storyboard file in Xcode. This canvas becomes your visual playground for laying out views and designing the flow of your app. - Adding Views and View Controllers:
Drag and drop views and view controllers onto the storyboard canvas. Connect them with segues to define the navigation flow between screens. - Configuring View Properties:
Interface Builder provides an intuitive interface for configuring view properties. Customize colors, fonts, and layout constraints directly in the storyboard.
UIKit Elements in Action: A Practical Example
Now, let's walk through a practical example to showcase how UIKit elements come together to build a simple iOS user interface. Assume we're creating a weather app with a main view displaying the current temperature and a button to refresh the data.
- Designing the Interface in Storyboard:
- Create a new view controller in the storyboard.
- Drag and drop a label for displaying the temperature and a button for refreshing data onto the view controller.
- Connect the label and button to the view controller's code using outlets.
- Coding the View Controller:
- Write Swift code to fetch weather data and update the UI. Connect the code to the storyboard using actions and outlets.
class WeatherViewController: UIViewController {
@IBOutlet weak var temperatureLabel: UILabel!
@IBAction func refreshButtonTapped(_ sender: UIButton) {
// Fetch weather data and update UI
let temperature = // Fetch temperature from API
temperatureLabel.text = "\(temperature)°C"
}
}
Here, the refreshButtonTapped
function is triggered when the user taps the refresh button. It fetches new weather data and updates the temperature label.
Responsive Layouts with Auto Layout: A UIKit Superpower
Creating user interfaces that adapt to different screen sizes and orientations is a critical aspect of iOS development. Auto Layout, another gem within UIKit, provides a constraint-based layout system to achieve responsive designs.
- Adding Constraints in Interface Builder:
- Use Interface Builder to add constraints to your views. Constraints define the relationship between views, specifying their positions and sizes relative to each other.
- Constraint Code in Swift:
- If you prefer, you can also define constraints programmatically in Swift code. This approach provides flexibility when dealing with dynamic UIs.
myButton.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activate([
myButton.topAnchor.constraint(equalTo: view.topAnchor, constant: 20),
myButton.leadingAnchor.constraint(equalTo: view.leadingAnchor, constant: 20),
myButton.widthAnchor.constraint(equalToConstant: 100),
myButton.heightAnchor.constraint(equalToConstant: 40)
])
Here, we anchor the top and leading edges of the button to the top and leading edges of the view, with specific constants to define the spacing.
UIKit Dynamics: Adding Life to User Interaction
UIKit Dynamics introduces physics-based animations and interactions, allowing your user interface elements to respond to gestures and movements in a natural and engaging manner. Here's a glimpse of how you can add dynamics to a view:
let animator = UIDynamicAnimator(referenceView: self.view)
let gravity = UIGravityBehavior(items: [myView])
animator.addBehavior(gravity)
In this example, the view myView
is affected by gravity, creating a dynamic and interactive experience.
Crafting Seamless iOS User Interfaces with UIKit
In conclusion, UIKit serves as the backbone for creating seamless and visually captivating user interfaces in iOS applications. From understanding the basics of views and view controllers to leveraging storyboards and Auto Layout for responsive designs, UIKit empowers developers to breathe life into their creative visions.
As you embark on your iOS app development journey, immerse yourself in the UIKit framework. Experiment with different views, explore the power of storyboards, and master Auto Layout to create user interfaces that not only meet but exceed user expectations. With UIKit as your ally, you have the tools to craft iOS applications that seamlessly blend form and function, providing users with an immersive and delightful experience. Happy coding, and may your UIKit adventures bring your app visions to life on the iOS canvas!